springBoot 文件下载
时间: 2023-07-25 19:27:32 浏览: 55
在Spring Boot中,您可以使用以下步骤来实现文件下载:
1. 在控制器类中定义一个处理文件下载请求的方法。例如:
```java
@GetMapping("/download")
public ResponseEntity<ByteArrayResource> downloadFile() {
// your code to read file content into a byte array
byte[] fileContent = ...;
// create a ByteArrayResource object from the byte array
ByteArrayResource resource = new ByteArrayResource(fileContent);
// set the headers for the response
HttpHeaders headers = new HttpHeaders();
headers.add(HttpHeaders.CONTENT_DISPOSITION, "attachment; filename=myfile.txt");
// create a ResponseEntity object with the resource and headers
return ResponseEntity.ok()
.headers(headers)
.contentLength(fileContent.length)
.contentType(MediaType.APPLICATION_OCTET_STREAM)
.body(resource);
}
```
在上面的示例中,我们从某个位置读取文件内容,并将其转换为一个`ByteArrayResource`对象。然后,我们设置响应头以指示浏览器将该文件作为附件下载,并创建一个`ResponseEntity`对象来包装该资源和响应头。
2. 在您的应用程序中配置一个`ResourceHandlerRegistry`,以便Spring Boot可以找到您要下载的文件。例如:
```java
@Override
public void addResourceHandlers(ResourceHandlerRegistry registry) {
registry.addResourceHandler("/files/**")
.addResourceLocations("file:~/myfiles/");
}
```
在上面的示例中,我们将请求路径`/files/**`映射到本地文件系统路径`~/myfiles/`。这意味着如果您的应用程序收到一个请求,其中路径以`/files/`开头,Spring Boot将尝试从本地文件系统中查找对应的文件。
如果您的文件不在本地文件系统中,而是在其他位置(例如Amazon S3或Google Cloud Storage),则可以使用Spring Boot提供的其他资源处理程序来实现。
3. 在前端页面中创建一个链接或按钮,以触发上面定义的文件下载请求。例如:
```html
<a href="/download">Download file</a>
```
在上面的示例中,我们创建了一个链接,将其指向上面定义的文件下载请求的路径`/download`。当用户单击此链接时,浏览器将发送一个HTTP GET请求到该路径,并下载文件作为附件。
这就是在Spring Boot中实现文件下载的基本步骤。请注意,您需要根据您的具体需求进行适当的调整和修改。
相关推荐
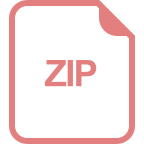













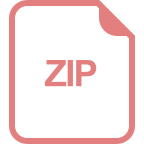