python好玩的代码
时间: 2023-11-12 10:13:17 浏览: 84
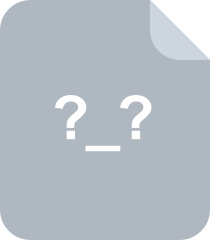
有趣的代码
以下是一些有趣的 Python 代码示例:
1. 生成随机密码
```python
import random
chars = 'abcdefghijklmnopqrstuvwxyz1234567890'
password = ''.join(random.choice(chars) for i in range(10))
print(password)
```
2. 猜数字游戏
```python
import random
secret_number = random.randint(1, 100)
print("I'm thinking of a number between 1 and 100.")
guess = int(input("Guess the number: "))
while guess != secret_number:
if guess < secret_number:
print("Too low.")
else:
print("Too high.")
guess = int(input("Guess again: "))
print("You guessed it! The number was", secret_number)
```
3. 打印彩虹
```python
colors = ['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'violet']
for color in colors:
print(color)
```
4. 生成斐波那契数列
```python
def fibonacci(n):
if n == 0:
return [0]
elif n == 1:
return [0, 1]
else:
fib = fibonacci(n-1)
fib.append(fib[-1] + fib[-2])
return fib
print(fibonacci(10))
```
5. 计算圆的面积和周长
```python
import math
radius = float(input("Enter the radius of the circle: "))
area = math.pi * radius**2
circumference = 2 * math.pi * radius
print("The area of the circle is", area)
print("The circumference of the circle is", circumference)
```
希望这些代码能给你带来一些乐趣!
阅读全文
相关推荐





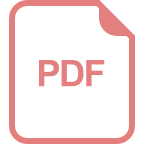








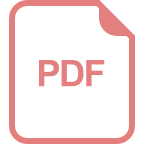