vue计算周数的时间戳
时间: 2023-07-26 21:36:05 浏览: 46
要计算某一时间戳是该年的第几周,可以使用 Vue.js 的 Moment.js 库来实现。具体代码如下:
```javascript
import moment from 'moment';
const timestamp = 1609459200000; // 2021年1月1日的时间戳
const weekNumber = moment(timestamp).isoWeek();
console.log(`该时间戳是第${weekNumber}周`);
```
这段代码中,我们首先引入了 Moment.js 库,并定义了一个时间戳 `timestamp`,它表示 2021 年 1 月 1 日的时间戳。然后,我们使用 Moment.js 的 `isoWeek()` 方法来计算该时间戳所在的 ISO 周数,最后将其输出到控制台中。这样,我们就可以得到该时间戳是该年的第几周了。
相关问题
vue 格式化时间戳
下面是Vue中格式化时间戳的方法:
1.在Vue中使用过滤器来格式化时间戳:
```javascript
Vue.filter('formatTime', function (value, format) {
const date = new Date(value * 1000);
const year = date.getFullYear();
const month = date.getMonth() + 1;
const day = date.getDate();
const hour = date.getHours();
const minute = date.getMinutes();
const second = date.getSeconds();
const padLeftZero = function (str) {
return ('00' + str).substr(str.length);
};
if (format === 'YYYY-MM-DD') {
return `${year}-${padLeftZero(month)}-${padLeftZero(day)}`;
} else if (format === 'YYYY-MM-DD HH:mm:ss') {
return `${year}-${padLeftZero(month)}-${padLeftZero(day)} ${padLeftZero(hour)}:${padLeftZero(minute)}:${padLeftZero(second)}`;
} else {
return '';
}
});
```
2.在Vue组件中使用过滤器来格式化时间戳:
```html
<template>
<div>
<p>{{ timestamp | formatTime('YYYY-MM-DD') }}</p>
<p>{{ timestamp | formatTime('YYYY-MM-DD HH:mm:ss') }}</p>
</div>
</template>
<script>
export default {
data() {
return {
timestamp: 1624452193
};
}
};
</script>
```
在上面的代码中,我们定义了一个名为`formatTime`的过滤器,它接受两个参数:时间戳和格式。然后,我们在Vue组件中使用这个过滤器来格式化时间戳。
vue获取当前时间戳
在Vue中获取当前时间戳可以使用JavaScript的`Date.now()`方法。在Vue组件中,你可以通过以下方式获取当前时间戳:
```javascript
new Date().getTime()
```
如果你想在Vue模板中获取当前时间戳,可以通过绑定一个计算属性的方式来实现:
```javascript
<template>
<div>{{ currentTime }}</div>
</template>
<script>
export default {
computed: {
currentTime() {
return Date.now();
}
}
}
</script>
```
这样,在模板中使用`{{ currentTime }}`将会显示当前的时间戳。
相关推荐
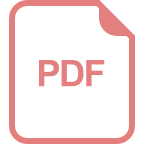
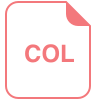











