编写一个简单的日程管理工具
时间: 2023-12-31 14:01:39 浏览: 35
好的,我可以为您提供以下实现步骤:
1. 设计需求:确定日程管理工具需要实现的基本功能,如添加、编辑、删除、查看日程等。
2. 数据库设计:根据需求设计数据库表结构,包括日程名称、时间、地点等字段。
3. 编写后端接口:使用一种后端语言(如Python、Java等)编写接口,提供前端调用,实现对数据库的增删改查操作。
4. 编写前端界面:使用一种前端框架(如React、Vue等)编写界面,与后端接口进行交互,实现对日程的管理和展示。
5. 测试和部署:对日程管理工具进行测试,修复问题,然后将其部署到服务器上,供用户使用。
以上是一个简单的日程管理工具的实现步骤,您可以根据自己的需求和技术水平进行修改和完善。
相关问题
python编写日程管理工具
好的,下面是一个简单的 Python 日程管理工具的代码示例:
```python
import datetime
def add_event(events):
event = {}
event["name"] = input("请输入事件名称:")
event_date = input("请输入事件日期 (YYYY-MM-DD):")
year, month, day = map(int, event_date.split("-"))
event["date"] = datetime.date(year, month, day)
event["time"] = input("请输入事件时间 (HH:MM):")
events.append(event)
def view_events(events):
if len(events) == 0:
print("无事件")
else:
for event in events:
print(event["date"].strftime("%Y-%m-%d"), event["time"], event["name"])
def main():
events = []
while True:
print("请选择操作:")
print("1. 添加事件")
print("2. 查看事件")
print("3. 退出")
choice = input()
if choice == "1":
add_event(events)
elif choice == "2":
view_events(events)
elif choice == "3":
break
else:
print("无效操作,请重新输入")
if __name__ == "__main__":
main()
```
这个程序使用了 Python 的 datetime 模块来处理日期和时间。它可以添加新事件,查看已存在的事件,并且可以退出程序。你可以根据自己的需求对其进行修改和扩展,比如增加删除事件的功能、将事件保存到文件中等等。
python编写一个简单的日程管理工具。包含添加日程,查询日程(按关键字查询),删除日程,查询日程列表(显示日程序号),编辑日程(按关键字显示原来的日程列表再编辑),退出程序的功能
好的,我可以帮您编写一个简单的日程管理工具。在这个工具中,我们将使用一个字典来存储所有的日程。在字典中,键是日程的编号,值是日程的内容。
以下是代码实现:
```python
schedule = {}
# 添加日程
def add_schedule():
schedule_id = input("请输入日程编号:")
schedule_text = input("请输入日程内容:")
schedule[schedule_id] = schedule_text
print("日程添加成功!")
# 查询日程
def search_schedule():
keyword = input("请输入关键字:")
for schedule_id, schedule_text in schedule.items():
if keyword in schedule_text:
print("编号:{},内容:{}".format(schedule_id, schedule_text))
# 删除日程
def delete_schedule():
schedule_id = input("请输入要删除的日程编号:")
if schedule_id in schedule:
del schedule[schedule_id]
print("日程删除成功!")
else:
print("日程不存在!")
# 查询日程列表
def list_schedule():
print("日程列表:")
for schedule_id in schedule:
print(schedule_id)
# 编辑日程
def edit_schedule():
keyword = input("请输入关键字:")
for schedule_id, schedule_text in schedule.items():
if keyword in schedule_text:
new_text = input("请输入新的日程内容:")
schedule[schedule_id] = new_text
print("日程修改成功!")
return
print("未找到符合条件的日程!")
# 主程序
while True:
print("请选择操作:")
print("1. 添加日程")
print("2. 查询日程")
print("3. 删除日程")
print("4. 查询日程列表")
print("5. 编辑日程")
print("6. 退出程序")
choice = input("请输入选项:")
if choice == "1":
add_schedule()
elif choice == "2":
search_schedule()
elif choice == "3":
delete_schedule()
elif choice == "4":
list_schedule()
elif choice == "5":
edit_schedule()
elif choice == "6":
break
else:
print("无效选项,请重新输入!")
```
在这个程序中,我们使用了一个 while 循环来不断接受用户的输入,并根据用户的选择来执行不同的操作。每个操作都对应一个函数,这些函数分别实现了添加、查询、删除、查询列表、编辑和退出功能。在这些函数中,我们使用了 input 函数来接受用户的输入,并使用 print 函数来输出结果。
请注意,这个程序只是一个简单的示例,可能还有很多需要改进的地方。例如,我们可以为每个日程添加更多的信息(如日期、时间等),或者使用数据库来存储日程信息。但是,这个程序已经足够演示如何使用 Python 来编写一个简单的日程管理工具了。
相关推荐
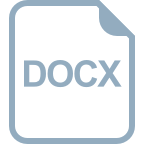
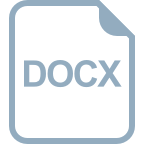












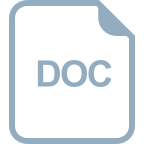