编写一个java类,可以根据用户id生成密钥,将明文转为密文,密文转为明文
时间: 2023-05-10 12:55:43 浏览: 114
好的,这是一个很好的编程问题。以下是一个简单的 Java 类,它可以根据用户 ID 生成密钥,将明文转换为密文,密文转换为明文:
```
import java.security.*;
import javax.crypto.*;
import javax.crypto.spec.*;
public class CryptoUtils {
private static final String ALGORITHM = "AES";
private static final String TRANSFORMATION = "AES/ECB/PKCS5Padding";
public static SecretKey generateKey(String userId) throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance(ALGORITHM);
SecureRandom secureRandom = new SecureRandom(userId.getBytes());
keyGenerator.init(128, secureRandom);
return keyGenerator.generateKey();
}
public static byte[] encrypt(SecretKey key, byte[] input) throws Exception {
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.ENCRYPT_MODE, key);
return cipher.doFinal(input);
}
public static byte[] decrypt(SecretKey key, byte[] input) throws Exception {
Cipher cipher = Cipher.getInstance(TRANSFORMATION);
cipher.init(Cipher.DECRYPT_MODE, key);
return cipher.doFinal(input);
}
}
```
这个类使用 AES 算法进行加密和解密。它包括三个方法:
- `generateKey`:根据用户 ID 生成一个密钥。
- `encrypt`:将明文转换为密文。
- `decrypt`:将密文转换为明文。
你可以使用这个类来加密和解密数据。例如,下面的代码演示了如何使用它:
```
String userId = "123456";
String plaintext = "Hello, world!";
SecretKey key = CryptoUtils.generateKey(userId);
byte[] ciphertext = CryptoUtils.encrypt(key, plaintext.getBytes());
byte[] decrypted = CryptoUtils.decrypt(key, ciphertext);
String decryptedText = new String(decrypted);
System.out.println(decryptedText);
```
这个代码片段将输出 "Hello, world!"。
阅读全文
相关推荐




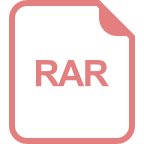











