用c语言实现dbscan算法
时间: 2023-05-21 10:01:59 浏览: 110
DBSCAN算法是一种基于密度的聚类算法,可以用于发现任意形状的簇。以下是用C语言实现DBSCAN算法的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_POINTS 1000
typedef struct {
double x, y;
} Point;
typedef struct {
int id;
int is_core;
int cluster_id;
} PointInfo;
int num_points;
Point points[MAX_POINTS];
PointInfo point_info[MAX_POINTS];
double eps;
int min_pts;
double distance(Point p1, Point p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
return sqrt(dx * dx + dy * dy);
}
int region_query(int p) {
int count = 0;
for (int i = 0; i < num_points; i++) {
if (i == p) continue;
if (distance(points[p], points[i]) <= eps) {
count++;
}
}
return count;
}
void expand_cluster(int p, int cluster_id) {
point_info[p].cluster_id = cluster_id;
int neighbor_pts[MAX_POINTS];
int num_neighbors = 0;
for (int i = 0; i < num_points; i++) {
if (i == p) continue;
if (distance(points[p], points[i]) <= eps) {
neighbor_pts[num_neighbors++] = i;
}
}
if (num_neighbors >= min_pts) {
point_info[p].is_core = 1;
for (int i = 0; i < num_neighbors; i++) {
int q = neighbor_pts[i];
if (point_info[q].cluster_id == -1) {
expand_cluster(q, cluster_id);
}
}
}
}
void dbscan() {
int cluster_id = 0;
for (int i = 0; i < num_points; i++) {
if (point_info[i].cluster_id != -1) continue;
if (region_query(i) >= min_pts) {
point_info[i].is_core = 1;
expand_cluster(i, cluster_id);
cluster_id++;
} else {
point_info[i].is_core = 0;
}
}
}
int main() {
// Read input data
scanf("%d %lf %d", &num_points, &eps, &min_pts);
for (int i = 0; i < num_points; i++) {
scanf("%lf %lf", &points[i].x, &points[i].y);
point_info[i].id = i;
point_info[i].is_core = -1;
point_info[i].cluster_id = -1;
}
// Run DBSCAN algorithm
dbscan();
// Print results
for (int i = 0; i < num_points; i++) {
printf("%d %d\n", point_info[i].id, point_info[i].cluster_id);
}
return 0;
}
```
该代码实现了一个简单的DBSCAN算法,可以读入点集数据并输出每个点所属的簇的编号。
相关推荐
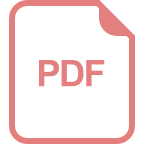
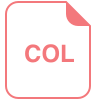
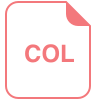
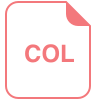
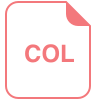
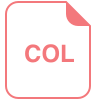









