用vue 的子传父父传子随机生成五道数学题
时间: 2023-07-12 22:47:06 浏览: 158
好的,我可以为您提供一个简单的实现,您可以根据您的需求进行修改和优化。
首先,我们需要在父组件中生成五个随机的数学题目,然后将这些题目传递给子组件。在子组件中,我们将这些题目显示出来,并且在用户完成答题后将结果传递回父组件。
以下是一个简单的实现,使用了Vue.js的props和emit来进行父子组件之间的数据传递:
父组件:
```html
<template>
<div>
<h2>请回答以下五道数学题:</h2>
<math-question v-for="(question, index) in questions" :key="index" :question="question" @answer="checkAnswer(index, $event)"></math-question>
</div>
</template>
<script>
import MathQuestion from './MathQuestion.vue'
export default {
components: {
MathQuestion
},
data() {
return {
questions: []
}
},
mounted() {
this.generateQuestions()
},
methods: {
generateQuestions() {
for (let i = 0; i < 5; i++) {
const num1 = Math.floor(Math.random() * 10)
const num2 = Math.floor(Math.random() * 10)
const operator = Math.random() > 0.5 ? '+' : '-'
const question = `${num1} ${operator} ${num2}`
this.questions.push(question)
}
},
checkAnswer(index, answer) {
// 检查用户答案是否正确
console.log(`第 ${index + 1} 道题,用户答案:${answer}`)
}
}
}
</script>
```
子组件:
```html
<template>
<div>
<p>{{ question }} = <input type="text" v-model="answer" @keydown.enter="submitAnswer"></p>
</div>
</template>
<script>
export default {
props: {
question: String
},
data() {
return {
answer: ''
}
},
methods: {
submitAnswer() {
this.$emit('answer', this.answer)
}
}
}
</script>
```
在父组件中,我们使用v-for指令来循环渲染五个MathQuestion组件,并将每个组件的题目通过props传递给它们。我们还为MathQuestion组件绑定了一个answer事件,以便在用户完成答题后检查答案。
在子组件中,我们使用v-model指令将输入框中的用户答案绑定到answer数据属性上。当用户按下回车键时,我们使用$emit方法触发answer事件,并将答案作为参数传递给父组件。
您可以根据您的需求进行修改和优化,例如添加更多的数学运算符,调整难度等级等。
阅读全文
相关推荐
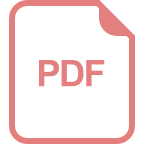
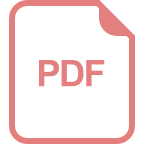





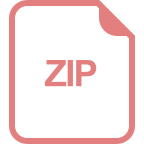
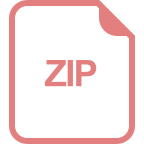
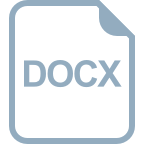
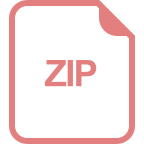
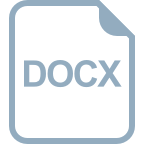
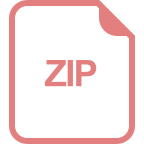