springboot +vue+elupload图片回显
时间: 2023-10-02 07:13:54 浏览: 148
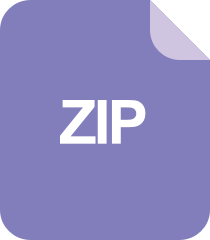
基于SpringBoot+Vue的图书管理系统.zip
首先,你需要在前端页面使用 el-upload 组件上传图片,并将图片的 base64 编码作为参数发送到后端。
然后,在后端使用 Spring Boot 编写接口,接收前端传来的图片的 base64 编码,将其解码成字节数组,再将字节数组转换成文件保存到服务器上。
在接口返回时,将图片的 URL 返回给前端,前端可以使用该 URL 在浏览器中显示图片。
下面是一个简单的示例代码:
前端代码:
```vue
<template>
<div>
<el-upload
class="upload-demo"
action="/uploadImage"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:headers="{ Authorization: 'Bearer ' + token }"
:data="{ userId: 123 }"
:show-file-list="false"
>
<el-button size="small" type="primary">点击上传</el-button>
</el-upload>
<img :src="imageUrl" v-if="imageUrl" />
</div>
</template>
<script>
export default {
data() {
return {
imageUrl: "",
token: "your_token",
};
},
methods: {
handleSuccess(response, file, fileList) {
this.imageUrl = response.url;
},
beforeUpload(file) {
const isJPG = file.type === "image/jpeg";
const isPNG = file.type === "image/png";
const isLt2M = file.size / 1024 / 1024 < 2;
if (!isJPG && !isPNG) {
this.$message.error("上传图片只能是 JPG/PNG 格式!");
return false;
}
if (!isLt2M) {
this.$message.error("上传图片大小不能超过 2MB!");
return false;
}
return true;
},
},
};
</script>
```
后端代码:
```java
@RestController
public class UploadController {
@PostMapping("/uploadImage")
public String uploadImage(@RequestParam("file") String base64Image, @RequestParam("userId") Long userId) throws IOException {
// 解码 base64 图片
String[] base64ImageParts = base64Image.split(",");
byte[] decodedBytes = Base64.getDecoder().decode(base64ImageParts[1]);
// 保存图片到服务器
String fileName = userId + "-" + UUID.randomUUID().toString() + ".jpg";
Path path = Paths.get("/path/to/save/" + fileName);
Files.write(path, decodedBytes);
// 返回图片 URL
return "http://your_domain.com/" + fileName;
}
}
```
注意,这只是一个简单的示例代码,你需要根据自己的实际需求进行适当的修改。
阅读全文
相关推荐
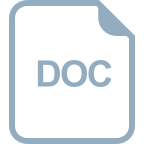
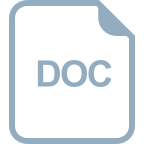
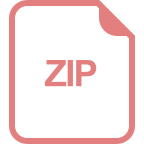
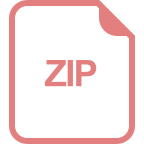
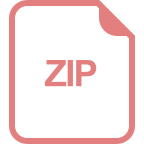
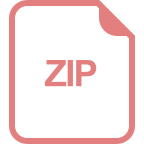
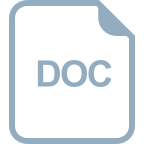
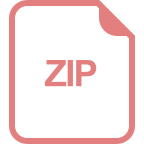
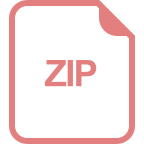
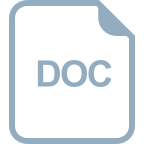
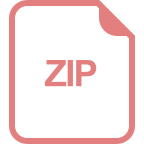
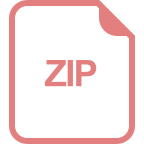
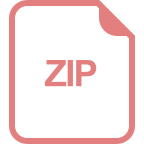
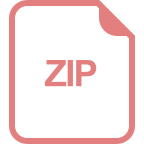
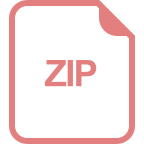
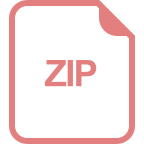
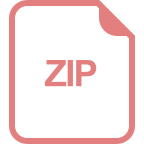
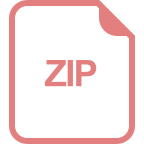