scikit-opt进行旅行商问题时,如何将某一条路线去除
时间: 2023-06-24 12:05:10 浏览: 75
如果您想要在 scikit-opt 中的旅行商问题(TSP)中去除某一条路线,可以使用以下步骤:
1. 首先,您需要定义您的旅行商问题。这可以通过创建一个包含城市坐标的矩阵来实现,例如:
```python
import numpy as np
from scipy.spatial.distance import cdist
# 定义城市坐标
coords = np.array([[1, 2], [3, 4], [5, 6], [7, 8]])
# 计算城市之间的距离
distances = cdist(coords, coords, metric='euclidean')
```
2. 接下来,您可以使用 scikit-opt 中的 `TSP` 类来解决旅行商问题,例如:
```python
from sko.GA import GA_TSP
# 定义旅行商问题
tsp = GA_TSP(func=lambda x: distances[np.concatenate([x, [x[0]]])].sum(), n_dim=len(coords))
# 运行遗传算法求解最优路径
best_path, best_distance = tsp.fit()
```
3. 如果您想要去掉某一条路径,可以在定义旅行商问题时添加一个额外的参数,例如:
```python
def tsp_without_path(path):
def func(x):
# 去掉指定的路径
x = np.delete(x, path)
# 计算总路径长度
return distances[np.concatenate([x, [x[0]]])].sum()
return func
# 定义旅行商问题(去掉第一条路径)
tsp = GA_TSP(func=tsp_without_path(0), n_dim=len(coords) - 1)
# 运行遗传算法求解最优路径
best_path, best_distance = tsp.fit()
```
在上述代码中,我们定义了一个新的函数 `tsp_without_path`,它接受一个要删除的路径索引,并返回一个新的函数,该函数计算总路径长度时会去掉指定的路径。然后,我们使用这个新函数来定义旅行商问题,并运行遗传算法来求解最优路径。
注意:如果您删除了某一条路径,那么最终得到的路径可能不是一个完美的回路,因为有可能存在无法连接的城市。因此,您可能需要另外编写一些代码来处理这种情况。
相关推荐
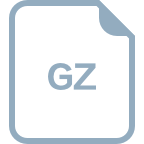
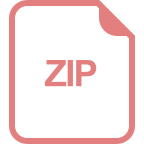
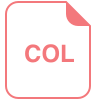
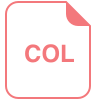
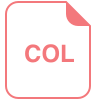
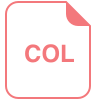
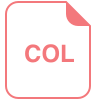









