写一个植物大战僵尸小游戏的c语言代码
时间: 2024-05-05 20:21:15 浏览: 199
以下是一个简单的植物大战僵尸小游戏的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define WIDTH 9
#define HEIGHT 5
#define SUNFLOWER_COST 50
#define PEASHOOTER_COST 100
#define ZOMBIE_HP 100
int board[HEIGHT][WIDTH] = {0};
int sun_count = 100;
void draw_board() {
int i, j;
printf("Sun Count: %d\n", sun_count);
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
switch (board[i][j]) {
case 0:
printf("_");
break;
case 1:
printf("S");
break;
case 2:
printf("P");
break;
case 3:
printf("Z");
break;
default:
printf("?");
break;
}
printf(" ");
}
printf("\n");
}
}
void place_sunflower() {
if (sun_count < SUNFLOWER_COST) {
printf("Not enough sun!\n");
return;
}
int row, col;
printf("Enter row and column to place sunflower: ");
scanf("%d %d", &row, &col);
if (row < 0 || row >= HEIGHT || col < 0 || col >= WIDTH) {
printf("Invalid position!\n");
return;
}
if (board[row][col] != 0) {
printf("Occupied!\n");
return;
}
board[row][col] = 1;
sun_count -= SUNFLOWER_COST;
}
void place_peashooter() {
if (sun_count < PEASHOOTER_COST) {
printf("Not enough sun!\n");
return;
}
int row, col;
printf("Enter row and column to place peashooter: ");
scanf("%d %d", &row, &col);
if (row < 0 || row >= HEIGHT || col < 0 || col >= WIDTH) {
printf("Invalid position!\n");
return;
}
if (board[row][col] != 0) {
printf("Occupied!\n");
return;
}
board[row][col] = 2;
sun_count -= PEASHOOTER_COST;
}
void generate_zombie() {
int row = rand() % HEIGHT;
board[row][WIDTH - 1] = 3;
}
void move_zombies() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = WIDTH - 1; j >= 0; j--) {
if (board[i][j] == 3) {
if (j == 0) {
printf("Game over!\n");
exit(0);
}
board[i][j] = 0;
board[i][j - 1] = 3;
}
}
}
}
void shoot_peas() {
int i, j;
for (i = 0; i < HEIGHT; i++) {
for (j = 0; j < WIDTH; j++) {
if (board[i][j] == 2) {
if (j == WIDTH - 1) {
printf("You win!\n");
exit(0);
}
if (board[i][j + 1] == 3) {
board[i][j + 1] = 0;
}
}
}
}
}
int main() {
srand(time(NULL));
while (1) {
draw_board();
int choice;
printf("1. Place sunflower\n");
printf("2. Place peashooter\n");
printf("3. End turn\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
place_sunflower();
break;
case 2:
place_peashooter();
break;
case 3:
generate_zombie();
move_zombies();
shoot_peas();
sun_count += 25;
break;
default:
printf("Invalid choice!\n");
break;
}
}
return 0;
}
```
这个代码实现了一个简单的控制台版本的植物大战僵尸游戏,玩家可以通过在网格上放置向日葵和豌豆射手来消灭僵尸,每回合结束时会生成新的僵尸并移动已有的僵尸。玩家需要在僵尸到达右侧边界之前尽可能多地消灭僵尸,否则游戏失败。玩家可以通过放置向日葵来增加阳光数量,阳光足够时可以放置豌豆射手。每个向日葵花费50阳光,每个豌豆射手花费100阳光,每回合结束会增加25阳光。
阅读全文
相关推荐
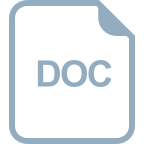
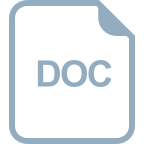
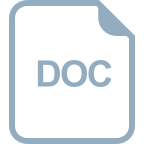
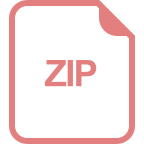
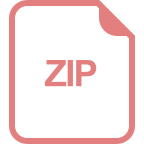
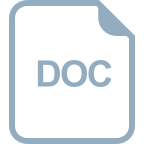
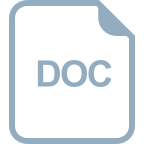




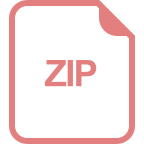
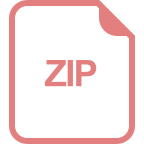
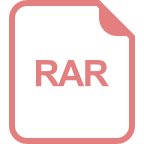
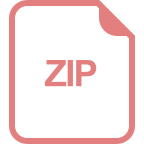
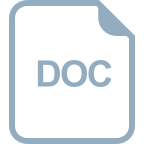

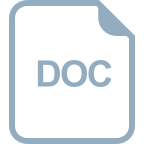
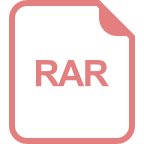