import java.util.ArrayList; import java.util.Collections; import java.util.Scanner; import java.util.Hashtable; public class Main{ public static void main(String[] args){ Scanner sc = new Scanner(System.in); int n = sc.nextInt(); Set set = new LinkedHashSet(); String a; String b = sc.nextLine(); for(int i =0 ;i <n;i++){ set.add(sc.nextLine()); } System.out.println(set.size()); for(Object obj:set){ System.out.println(obj); } sc.close(); } }
时间: 2024-04-27 22:22:57 浏览: 10
这段代码实现了从标准输入中读取n个字符串,并去重后输出不重复字符串个数和每个字符串。具体实现过程如下:
1. 导入需要使用的包,包括`java.util.Scanner`、`java.util.Set`和`java.util.LinkedHashSet`。
2. 创建一个`Scanner`对象`sc`,用于从标准输入中读取数据。
3. 从标准输入中读取一个整数`n`,表示接下来要读取的字符串个数。
4. 创建一个`LinkedHashSet`对象`set`,用于存储不重复的字符串。
5. 使用`for`循环,循环读取`n`个字符串,并将其添加到`set`中。
6. 输出不重复字符串的个数,即`set`的大小。
7. 使用`for`循环遍历`set`中的每个元素,输出每个字符串。
8. 关闭`Scanner`对象。
这段代码中使用了`LinkedHashSet`来实现去重功能,与`HashSet`相比,`LinkedHashSet`能够保持元素的插入顺序,因此在输出时能够保证按照输入顺序输出。另外,由于`LinkedHashSet`继承自`HashSet`,因此也具有去重的功能。
相关问题
import java.util.Scanner;
As an AI language model, I cannot perform input/output operations or run Java code. However, here is an example of how the Scanner class can be used in Java to read user input from the console:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter your name: ");
String name = scanner.nextLine();
System.out.println("Hello, " + name + "!");
scanner.close();
}
}
```
In this example, we create a Scanner object to read input from the console using `System.in`. We then prompt the user to enter their name and read their input using the `nextLine()` method of the Scanner class. Finally, we print out a greeting using the user's name. Note that we close the Scanner object at the end to prevent resource leaks.
import java.util.Scanner什么意思
这是Java语言中的一个类Scanner,用于读取用户输入的数据。可以使用Scanner类的next()、nextInt()、nextDouble()等方法来读取不同类型的数据。例如,下面的代码可以读取用户输入的字符串并输出:
```
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入一个字符串:");
String str = scanner.next();
System.out.println("您输入的字符串是:" + str);
}
}
```
在上面的代码中,首先使用import语句导入Scanner类,然后创建一个Scanner对象scanner,使用next()方法读取用户输入的字符串,最后输出读取到的字符串。
相关推荐
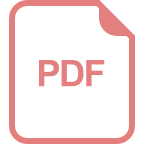
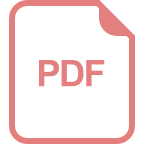













