C语言结构体变量在外部怎么赋值
时间: 2023-06-22 22:37:49 浏览: 221
在外部为C语言结构体变量赋值,可以通过以下两种方法实现:
1. 使用点运算符直接为结构体变量中的成员赋值。例如:
```
struct student {
char name[20];
int age;
float score;
};
struct student stu1;
stu1.age = 18; // 为结构体变量stu1的age成员赋值
```
2. 使用结构体指针来为结构体变量赋值。例如:
```
struct student {
char name[20];
int age;
float score;
};
struct student stu1, *p;
p = &stu1; // 将结构体变量stu1的地址赋给指针p
(*p).age = 18; // 通过指针p为结构体变量stu1的age成员赋值
```
其中,第二种方法也可以使用箭头运算符来简化语法,例如:
```
p->age = 18; // 通过指针p为结构体变量stu1的age成员赋值
```
相关问题
c语言结构体中指针变量在外部函数动态分配内存
在C语言中,结构体中的指针变量可以在外部函数中动态分配内存。具体实现方法如下:
1. 定义一个结构体,其中包含一个指针变量。
2. 在外部函数中,使用malloc函数动态分配内存,并将分配的内存地址赋值给结构体中的指针变量。
3. 在使用完毕后,需要使用free函数释放内存。
示例代码如下:
```
#include <stdio.h>
#include <stdlib.h>
struct student {
char name[20];
int age;
int *score;
};
void allocate_memory(struct student *stu) {
stu->score = (int*)malloc(sizeof(int) * 3);
if (stu->score == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
}
int main() {
struct student stu;
allocate_memory(&stu);
stu.score[] = 90;
stu.score[1] = 80;
stu.score[2] = 85;
printf("Name: %s\n", stu.name);
printf("Age: %d\n", stu.age);
printf("Score: %d %d %d\n", stu.score[], stu.score[1], stu.score[2]);
free(stu.score);
return ;
}
```
在上面的示例代码中,我们定义了一个结构体student,其中包含一个指针变量score。在外部函数allocate_memory中,我们使用malloc函数动态分配了3个int类型的内存空间,并将分配的内存地址赋值给stu.score。在主函数中,我们可以通过stu.score来访问分配的内存空间,并对其进行操作。最后,我们需要使用free函数释放内存空间。
c语言结构体
C语言中的结构体是一种自定义的数据类型,它可以包含多个不同类型的变量,这些变量被称为结构体成员。结构体的定义通常放在函数外部,在需要使用结构体的函数内部可以声明结构体变量。
结构体的定义格式如下:
```
struct struct_name {
data_type member1;
data_type member2;
...
data_type memberN;
};
```
其中,`struct_name` 是结构体的名称,`member1` 到 `memberN` 是结构体的成员,每个成员都有自己的数据类型。
例如,我们可以定义一个表示学生信息的结构体如下:
```
struct student {
char name[20];
int age;
float score;
};
```
这个结构体包含三个成员:姓名、年龄和分数,它们分别是字符型数组、整型和浮点型。
在函数内部声明结构体变量的语法如下:
```
struct struct_name variable_name;
```
例如,我们可以在主函数中声明一个名为 `stu` 的 `student` 类型的结构体变量:
```
int main() {
struct student stu;
...
return 0;
}
```
这个结构体变量可以像普通变量一样使用,例如:
```
strcpy(stu.name, "Tom");
stu.age = 18;
stu.score = 90.5;
```
以上代码给 `stu` 结构体变量的成员赋值。
我们也可以用指向结构体的指针访问结构体成员,例如:
```
struct student *pstu = &stu;
strcpy(pstu->name, "Tom");
pstu->age = 18;
pstu->score = 90.5;
```
以上代码用指针 `pstu` 访问 `stu` 结构体变量的成员赋值,其中 `->` 是结构体指针运算符。
这就是C语言中结构体的基本用法。
阅读全文
相关推荐
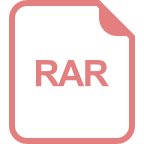
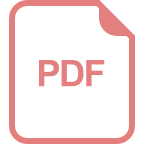
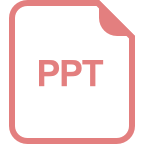
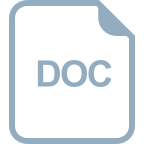
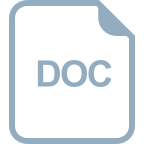
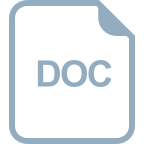
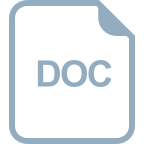




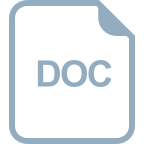
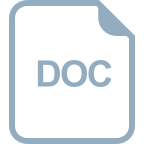
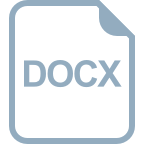
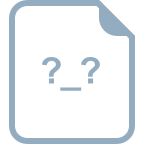
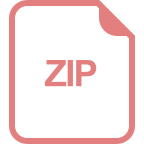
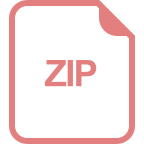
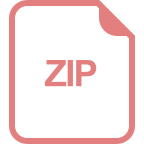