磁盘调度算法Java代码,使用FCFS,CSCAN,SCAN,SSTF算法
时间: 2023-09-17 14:09:55 浏览: 88
下面是使用Java实现FCFS、CSCAN、SCAN、SSTF算法的磁盘调度代码:
```java
import java.util.*;
public class DiskScheduling {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.print("Enter the head position: ");
int head = sc.nextInt();
System.out.print("Enter the number of disk requests: ");
int n = sc.nextInt();
int[] requests = new int[n];
System.out.println("Enter the disk requests: ");
for (int i = 0; i < n; i++) {
requests[i] = sc.nextInt();
}
// FCFS
int[] fcfsSequence = new int[n + 1];
fcfsSequence[0] = head;
for (int i = 0; i < n; i++) {
fcfsSequence[i + 1] = requests[i];
}
System.out.println("FCFS: " + Arrays.toString(fcfsSequence));
// CSCAN
int[] cscanSequence = new int[n + 2];
cscanSequence[0] = head;
cscanSequence[n + 1] = 0;
int[] cscanRequests = new int[n + 2];
cscanRequests[0] = head;
cscanRequests[n + 1] = 0;
for (int i = 0; i < n; i++) {
cscanRequests[i + 1] = requests[i];
}
Arrays.sort(cscanRequests);
int index = Arrays.binarySearch(cscanRequests, head);
if (index < 0) {
index = -index - 1;
}
int j = 1;
for (int i = index; i <= n + 1; i++, j++) {
cscanSequence[j] = cscanRequests[i];
}
for (int i = 1; i < index; i++, j++) {
cscanSequence[j] = cscanRequests[i];
}
System.out.println("CSCAN: " + Arrays.toString(cscanSequence));
// SCAN
int[] scanSequence = new int[n + 1];
scanSequence[0] = head;
int[] scanRequests = new int[n + 1];
for (int i = 0; i < n; i++) {
scanRequests[i] = requests[i];
}
Arrays.sort(scanRequests);
index = Arrays.binarySearch(scanRequests, head);
if (index < 0) {
index = -index - 1;
}
int i = index - 1, j = index + 1, k = 1;
while (i >= 0 && j < n) {
if (head - scanRequests[i] < scanRequests[j] - head) {
scanSequence[k++] = scanRequests[i--];
} else {
scanSequence[k++] = scanRequests[j++];
}
}
while (i >= 0) {
scanSequence[k++] = scanRequests[i--];
}
while (j < n) {
scanSequence[k++] = scanRequests[j++];
}
System.out.println("SCAN: " + Arrays.toString(scanSequence));
// SSTF
int[] sstfSequence = new int[n + 1];
sstfSequence[0] = head;
int[] sstfRequests = new int[n];
for (int x = 0; x < n; x++) {
sstfRequests[x] = requests[x];
}
for (int x = 0; x < n; x++) {
int min = Integer.MAX_VALUE;
int minIndex = -1;
for (int y = 0; y < n - x; y++) {
if (Math.abs(head - sstfRequests[y]) < min) {
min = Math.abs(head - sstfRequests[y]);
minIndex = y;
}
}
sstfSequence[x + 1] = sstfRequests[minIndex];
head = sstfRequests[minIndex];
sstfRequests[minIndex] = sstfRequests[n - x - 1];
}
System.out.println("SSTF: " + Arrays.toString(sstfSequence));
}
}
```
这个程序实现了FCFS、CSCAN、SCAN、SSTF算法的磁盘调度。用户可以输入磁头的初始位置、磁盘请求的数量和请求的位置。程序使用不同的算法对磁盘进行扫描,并输出磁头访问的顺序。其中,FCFS算法按照请求的顺序进行扫描;CSCAN算法按照磁头移动的方向扫描磁盘,并在两端循环;SCAN算法按照磁头移动的方向扫描磁盘,直到到达最边缘,然后返回扫描另一侧的磁盘;SSTF算法选择最近的磁盘访问。
阅读全文
相关推荐
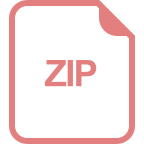
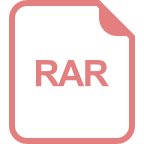
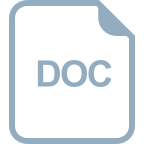
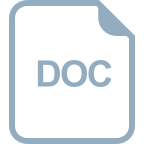
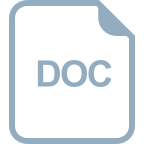
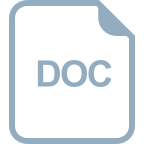
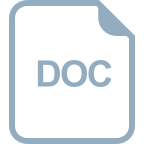
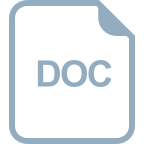
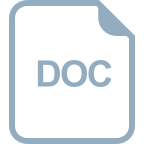

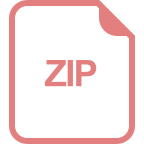
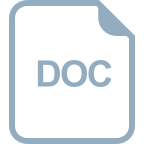
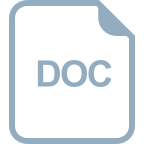
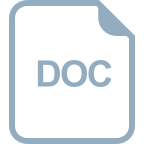

