opencv图像旋转动画
时间: 2023-09-14 12:11:30 浏览: 118
要在 OpenCV 中实现图像旋转动画,可以使用以下步骤:
1. 加载图像并创建一个空白帧。
2. 定义旋转中心点和旋转角度。
3. 使用 `cv2.getRotationMatrix2D()` 函数创建旋转矩阵。
4. 使用 `cv2.warpAffine()` 函数将图像旋转到指定角度。
5. 将旋转后的图像添加到空白帧中。
6. 显示动画。
下面是一个示例代码,其中旋转角度每秒递增1度,直到旋转到360度即完整一圈。
```python
import cv2
import numpy as np
# 加载图像
img = cv2.imread('image.jpg')
# 创建空白帧
h, w = img.shape[:2]
blank_frame = np.zeros((h, w, 3), dtype=np.uint8)
# 定义旋转中心点和初始旋转角度
center = (w//2, h//2)
angle = 0
# 动画循环
while True:
# 创建旋转矩阵
M = cv2.getRotationMatrix2D(center, angle, 1.0)
# 将图像旋转到指定角度
rotated_img = cv2.warpAffine(img, M, (w, h))
# 将旋转后的图像添加到空白帧中
blank_frame = cv2.addWeighted(blank_frame, 0.5, rotated_img, 0.5, 0)
# 显示动画
cv2.imshow('Rotating Image Animation', blank_frame)
cv2.waitKey(10)
# 角度递增
angle += 1
# 如果旋转角度超过360度,重新开始旋转
if angle == 360:
angle = 0
```
运行代码后,会出现一个显示动画的窗口,其中图像会不断旋转。可以按下 ESC 键来退出动画。
阅读全文
相关推荐
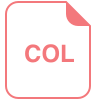
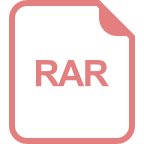
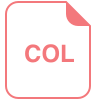
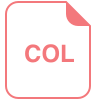
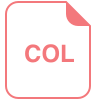
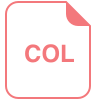
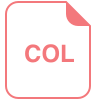
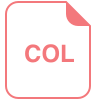
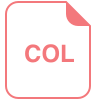
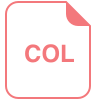
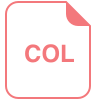
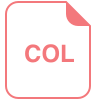
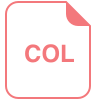
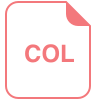
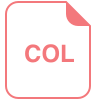
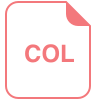