vue3+typescripts表格某一列匹配字典
时间: 2023-07-22 17:58:45 浏览: 55
要在Vue 3和TypeScript中实现表格某一列的字典匹配,你可以按照以下步骤进行操作:
1. 首先,确保你已经安装了Vue 3和TypeScript,以及相关的依赖。
2. 在Vue组件中定义表格数据和字典数据。你可以使用`ref`来声明响应式的变量,并将其初始化为空数组或空对象。
```typescript
import { defineComponent, ref } from 'vue';
export default defineComponent({
setup() {
const tableData = ref([]);
const dictionaryData = ref({});
// 获取表格数据和字典数据的方法,可以从后端接口获取或其他方式
const fetchTableData = () => {
// ...
};
const fetchDictionaryData = () => {
// ...
};
// 在组件初始化时获取表格数据和字典数据
fetchTableData();
fetchDictionaryData();
return {
tableData,
dictionaryData,
}
},
});
```
3. 在获取到表格数据和字典数据后,你可以使用计算属性来进行字典匹配,并返回处理后的表格数据。
```typescript
import { computed } from 'vue';
// ...
export default defineComponent({
// ...
computed: {
processedTableData() {
return computed(() => {
return this.tableData.value.map((row: any) => {
const gender = row.gender;
const matchedValue = this.dictionaryData.value[gender] || '';
return { ...row, gender: matchedValue };
});
});
},
},
});
```
4. 在模板中渲染处理后的表格数据。
```html
<template>
<table>
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Gender</th>
</tr>
</thead>
<tbody>
<tr v-for="row in processedTableData" :key="row.id">
<td>{{ row.id }}</td>
<td>{{ row.name }}</td>
<td>{{ row.gender }}</td>
</tr>
</tbody>
</table>
</template>
```
通过以上步骤,你可以在Vue 3和TypeScript中实现表格某一列的字典匹配。请根据实际情况修改示例代码中的变量名、数据获取方法和具体逻辑。
相关推荐
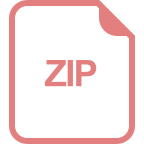
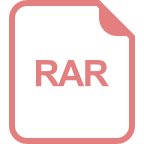
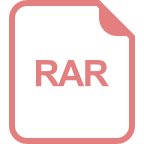














