帮我用android studio 代码实现安卓播放器界面
时间: 2023-07-20 08:34:35 浏览: 117
好的,以下是一个简单的安卓视频播放器界面的实现代码示例:
1. 首先,在Android Studio中创建一个新的安卓项目,并在布局文件中添加以下代码:
```
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<VideoView
android:id="@+id/videoView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<LinearLayout
android:id="@+id/controlsLayout"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:orientation="horizontal"
android:padding="10dp">
<ImageButton
android:id="@+id/playButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_play_arrow_black_24dp" />
<SeekBar
android:id="@+id/seekBar"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_weight="1"
android:paddingLeft="5dp"
android:paddingRight="5dp" />
<TextView
android:id="@+id/timeTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="00:00"
android:textAppearance="?android:attr/textAppearanceSmall" />
<ImageButton
android:id="@+id/fullScreenButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_fullscreen_black_24dp" />
</LinearLayout>
</RelativeLayout>
```
2. 在Java代码中,您需要为播放器控件添加一些基本操作,例如播放、暂停、拖动进度条、切换全屏等。以下是一个简单的示例代码:
```
public class VideoPlayerActivity extends AppCompatActivity {
private VideoView videoView;
private ImageButton playButton, fullScreenButton;
private SeekBar seekBar;
private TextView timeTextView;
private boolean isFullScreen = false;
private boolean isPlaying = false;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_video_player);
// 获取控件
videoView = (VideoView) findViewById(R.id.videoView);
playButton = (ImageButton) findViewById(R.id.playButton);
fullScreenButton = (ImageButton) findViewById(R.id.fullScreenButton);
seekBar = (SeekBar) findViewById(R.id.seekBar);
timeTextView = (TextView) findViewById(R.id.timeTextView);
// 设置视频路径
videoView.setVideoPath("YOUR_VIDEO_PATH");
// 监听视频播放完成事件
videoView.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mediaPlayer) {
isPlaying = false;
playButton.setImageResource(R.drawable.ic_play_arrow_black_24dp);
seekBar.setProgress(0);
timeTextView.setText("00:00");
}
});
// 监听播放按钮点击事件
playButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (isPlaying) {
videoView.pause();
isPlaying = false;
playButton.setImageResource(R.drawable.ic_play_arrow_black_24dp);
} else {
videoView.start();
isPlaying = true;
playButton.setImageResource(R.drawable.ic_pause_black_24dp);
}
}
});
// 监听全屏按钮点击事件
fullScreenButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
if (isFullScreen) {
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_PORTRAIT);
isFullScreen = false;
fullScreenButton.setImageResource(R.drawable.ic_fullscreen_black_24dp);
} else {
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
isFullScreen = true;
fullScreenButton.setImageResource(R.drawable.ic_fullscreen_exit_black_24dp);
}
}
});
// 监听进度条拖动事件
seekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
if (fromUser) {
videoView.seekTo(progress * 1000);
timeTextView.setText(getFormattedTime(progress));
}
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
videoView.pause();
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
videoView.start();
}
});
// 开始播放视频
videoView.start();
isPlaying = true;
playButton.setImageResource(R.drawable.ic_pause_black_24dp);
// 设置进度条最大值
seekBar.setMax(videoView.getDuration() / 1000);
// 更新进度条和时间显示
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
if (isPlaying) {
seekBar.setProgress(videoView.getCurrentPosition() / 1000);
timeTextView.setText(getFormattedTime(videoView.getCurrentPosition() / 1000));
}
new Handler().postDelayed(this, 1000);
}
}, 1000);
}
// 格式化时间,将秒数转换为00:00格式
private String getFormattedTime(int seconds) {
int minutes = seconds / 60;
seconds = seconds % 60;
return String.format("%02d:%02d", minutes, seconds);
}
}
```
这些代码将实现一个简单的安卓视频播放器界面,包括视频播放、进度条拖动、暂停/播放、全屏切换等功能。当然,您需要将“YOUR_VIDEO_PATH”替换为您实际视频文件的路径。
希望这个示例代码可以帮助您实现一个基本的安卓视频播放器界面。
阅读全文
相关推荐




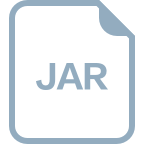










