windows下通过qt使用zlib将一个文件夹压缩成.zip后并保留文件夹内部文件的后缀
时间: 2024-02-05 11:12:03 浏览: 23
你可以使用zlib库提供的函数来实现将一个文件夹压缩成.zip的功能,同时保留文件夹内部文件的后缀。以下是一个简单的示例代码:
```c++
#include <QtCore>
#include <QDebug>
#include <zlib.h>
#define CHUNK_SIZE 16384
bool compressDirectory(const QString &sourcePath, const QString &destinationPath)
{
QFile destinationFile(destinationPath);
if (!destinationFile.open(QIODevice::WriteOnly))
return false;
gzFile destination = gzopen(destinationPath.toUtf8().constData(), "wb");
if (!destination)
return false;
QFileInfo sourceInfo(sourcePath);
if (!sourceInfo.isDir())
return false;
QFileInfoList files = QDir(sourcePath).entryInfoList(QDir::Files | QDir::Dirs | QDir::NoDotAndDotDot, QDir::Name);
foreach (const QFileInfo &file, files) {
QString filePath = file.absoluteFilePath();
QString fileName = file.fileName();
QString relativePath = filePath.mid(sourcePath.length());
if (file.isDir()) {
gzprintf(destination, "%s%s\n", qPrintable(relativePath), "/");
} else {
QFile sourceFile(filePath);
if (!sourceFile.open(QIODevice::ReadOnly))
return false;
QByteArray buffer;
while (!sourceFile.atEnd()) {
buffer = sourceFile.read(CHUNK_SIZE);
gzwrite(destination, buffer.constData(), buffer.size());
}
gzprintf(destination, "%s\n", qPrintable(relativePath));
}
}
gzclose(destination);
return true;
}
```
这段代码使用了zlib库提供的gzFile结构体和相关函数来将数据写入压缩文件。在这里,我们使用了gzprintf()函数来向压缩文件中写入数据。对于文件夹,我们将其名称加上一个斜杠(/)后写入压缩文件中,对于文件则直接写入其相对路径和文件名。
你可以在Qt的应用程序中调用这个函数来实现将一个文件夹压缩成.zip的功能,并保留文件夹内部文件的后缀。
相关推荐
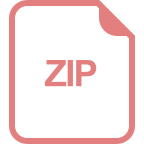
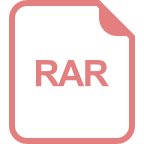











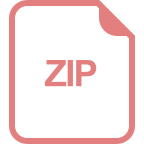