在Android开发中使用Java编写,如何实现一个按钮的左滑出现删除及评价选项的效果呢?可以帮我写出一个详细的布局文件嘛
时间: 2024-05-14 19:16:15 浏览: 12
可以使用RecyclerView和ItemTouchHelper来实现一个按钮左滑出现删除及评价选项的效果,以下是一个简单的示例布局文件:
```xml
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_margin="8dp"
android:background="@color/white"
android:clipToPadding="false"
android:padding="8dp"
app:layoutManager="androidx.recyclerview.widget.LinearLayoutManager"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent" />
```
在代码中使用ItemTouchHelper和ItemTouchHelper.SimpleCallback来处理滑动事件,例如:
```java
ItemTouchHelper.SimpleCallback simpleCallback = new ItemTouchHelper.SimpleCallback(0,
ItemTouchHelper.LEFT | ItemTouchHelper.RIGHT) {
@Override
public boolean onMove(@NonNull RecyclerView recyclerView, @NonNull RecyclerView.ViewHolder viewHolder, @NonNull RecyclerView.ViewHolder target) {
return false;
}
@Override
public void onSwiped(@NonNull RecyclerView.ViewHolder viewHolder, int direction) {
int position = viewHolder.getAdapterPosition();
if (direction == ItemTouchHelper.LEFT) {
// 左滑出现删除选项
adapter.removeItem(position);
} else if (direction == ItemTouchHelper.RIGHT) {
// 右滑出现评价选项
adapter.showRatingDialog(position);
}
}
@Override
public void onChildDraw(@NonNull Canvas c, @NonNull RecyclerView recyclerView, @NonNull RecyclerView.ViewHolder viewHolder,
float dX, float dY, int actionState, boolean isCurrentlyActive) {
View itemView = viewHolder.itemView;
if (actionState == ItemTouchHelper.ACTION_STATE_SWIPE) {
// 左滑距离
float swipeThreshold = itemView.getWidth() * 0.3f;
// 删除和评价按钮的宽度
float buttonWidth = itemView.getWidth() * 0.3f;
if (dX < -swipeThreshold) {
// 左滑超过阈值,显示删除按钮
drawDeleteButton(c, itemView, dX, buttonWidth);
} else if (dX > swipeThreshold) {
// 右滑超过阈值,显示评价按钮
drawRatingButton(c, itemView, dX, buttonWidth);
}
}
super.onChildDraw(c, recyclerView, viewHolder, dX, dY, actionState, isCurrentlyActive);
}
private void drawDeleteButton(Canvas c, View itemView, float dX, float buttonWidth) {
Paint paint = new Paint();
paint.setColor(Color.RED);
RectF deleteButton = new RectF(itemView.getRight() + dX - buttonWidth, itemView.getTop(),
itemView.getRight() + dX, itemView.getBottom());
c.drawRect(deleteButton, paint);
float textSize = TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 16,
getResources().getDisplayMetrics());
paint.setTextSize(textSize);
paint.setColor(Color.WHITE);
String deleteText = "删除";
Rect textBounds = new Rect();
paint.getTextBounds(deleteText, 0, deleteText.length(), textBounds);
c.drawText(deleteText, deleteButton.centerX() - textBounds.width() / 2f,
deleteButton.centerY() + textBounds.height() / 2f, paint);
}
private void drawRatingButton(Canvas c, View itemView, float dX, float buttonWidth) {
Paint paint = new Paint();
paint.setColor(Color.BLUE);
RectF ratingButton = new RectF(itemView.getLeft(), itemView.getTop(),
itemView.getLeft() + buttonWidth, itemView.getBottom());
c.drawRect(ratingButton, paint);
float textSize = TypedValue.applyDimension(TypedValue.COMPLEX_UNIT_SP, 16,
getResources().getDisplayMetrics());
paint.setTextSize(textSize);
paint.setColor(Color.WHITE);
String ratingText = "评价";
Rect textBounds = new Rect();
paint.getTextBounds(ratingText, 0, ratingText.length(), textBounds);
c.drawText(ratingText, ratingButton.centerX() - textBounds.width() / 2f,
ratingButton.centerY() + textBounds.height() / 2f, paint);
}
};
ItemTouchHelper itemTouchHelper = new ItemTouchHelper(simpleCallback);
itemTouchHelper.attachToRecyclerView(recyclerView);
```
这个示例布局文件和代码可以帮助你实现一个简单的左滑出现删除及评价选项的效果,但具体实现还需要根据你的具体需求进行调整。
相关推荐
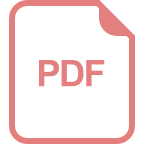
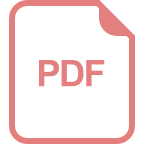














