请帮我编写一个基于逻辑回归原理的python代码
时间: 2024-03-17 08:47:24 浏览: 62
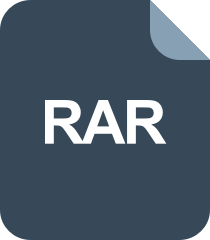
逻辑回归python代码
好的,以下是一个基于逻辑回归原理的Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成样本数据
np.random.seed(0)
X = np.random.randn(100, 2)
Y = np.logical_xor(X[:, 0] > 0, X[:, 1] > 0)
# 绘制样本数据散点图
plt.scatter(X[:, 0], X[:, 1], c=Y, s=40, cmap=plt.cm.Spectral)
plt.show()
# 定义逻辑回归模型
class LogisticRegression:
def __init__(self, learning_rate=0.1, num_iterations=10000):
self.learning_rate = learning_rate
self.num_iterations = num_iterations
def sigmoid(self, z):
return 1 / (1 + np.exp(-z))
def initialize_weights(self, n_features):
self.w = np.zeros((n_features, 1))
self.b = 0
def forward_propagation(self, X):
z = np.dot(X, self.w) + self.b
a = self.sigmoid(z)
return a
def compute_cost(self, a, Y):
m = Y.shape[0]
cost = (-1/m) * np.sum(Y * np.log(a) + (1-Y) * np.log(1-a))
return cost
def backward_propagation(self, X, Y, a):
m = Y.shape[0]
dz = a - Y
dw = (1/m) * np.dot(X.T, dz)
db = (1/m) * np.sum(dz)
return dw, db
def update_weights(self, dw, db):
self.w = self.w - self.learning_rate * dw
self.b = self.b - self.learning_rate * db
def train(self, X, Y):
n_features = X.shape[1]
self.initialize_weights(n_features)
for i in range(self.num_iterations):
a = self.forward_propagation(X)
cost = self.compute_cost(a, Y)
dw, db = self.backward_propagation(X, Y, a)
self.update_weights(dw, db)
if i % 1000 == 0:
print("Cost after iteration %i: %f" % (i, cost))
def predict(self, X):
a = self.forward_propagation(X)
Y_predict = np.round(a)
return Y_predict
# 训练逻辑回归模型
model = LogisticRegression(learning_rate=0.1, num_iterations=10000)
model.train(X, Y)
# 绘制决策边界
x1_min, x1_max = X[:, 0].min() - 0.1, X[:, 0].max() + 0.1
x2_min, x2_max = X[:, 1].min() - 0.1, X[:, 1].max() + 0.1
xx1, xx2 = np.meshgrid(np.linspace(x1_min, x1_max, 100), np.linspace(x2_min, x2_max, 100))
Z = model.predict(np.c_[xx1.ravel(), xx2.ravel()])
Z = Z.reshape(xx1.shape)
plt.contourf(xx1, xx2, Z, cmap=plt.cm.Spectral, alpha=0.8)
plt.scatter(X[:, 0], X[:, 1], c=Y, s=40, cmap=plt.cm.Spectral)
plt.show()
```
这个代码示例中,我们首先生成了一个逻辑异或的样本数据集,然后使用逻辑回归模型进行训练,最终绘制出了决策边界。其中,逻辑回归模型的实现包括了前向传播、反向传播和参数更新等步骤。
阅读全文
相关推荐
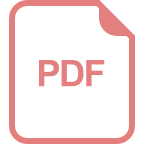
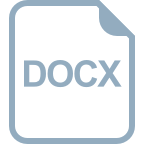


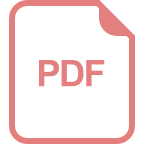
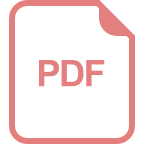
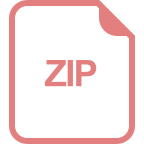
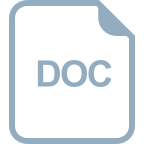
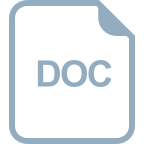
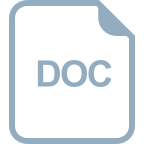
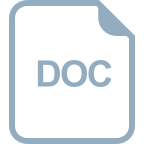
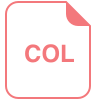



