用c++写该题的具体代码 以班级为单位进行选题,题目信息以文件形式存放, 包括:编号、题目、任务要求、完成时间 功能要求: 1学生信息包括:学号、姓名、班级; 2输入学号,随机抽取题目,限定每个题目只能被一个学生选中 3选题结果以文件形式存放,可以随时进行查询操作。
时间: 2024-02-23 11:56:30 浏览: 22
好的,以下是用 C++ 实现该题的代码:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <string>
#include <ctime>
#include <cstdlib>
using namespace std;
struct Student {
string id; // 学号
string name; // 姓名
string cls; // 班级
};
struct Topic {
string id; // 编号
string title; // 题目
string requirement; // 任务要求
string deadline; // 完成时间
string studentId; // 学生学号,初始值为空字符串
};
vector<Student> students; // 学生列表
vector<Topic> topics; // 题目列表
vector<Topic> selections; // 选题结果
// 读取学生信息
void readStudents() {
ifstream fin("students.txt");
string id, name, cls;
while (fin >> id >> name >> cls) {
students.push_back({id, name, cls});
}
fin.close();
}
// 读取题目信息
void readTopics() {
ifstream fin("topics.txt");
string id, title, requirement, deadline;
while (fin >> id >> title >> requirement >> deadline) {
topics.push_back({id, title, requirement, deadline, ""});
}
fin.close();
}
// 随机抽取题目
Topic* selectTopic(const string& studentId) {
vector<Topic*> availableTopics;
for (auto& topic : topics) {
if (topic.studentId.empty()) {
availableTopics.push_back(&topic);
}
}
if (availableTopics.empty()) {
return nullptr;
}
srand(time(nullptr));
int index = rand() % availableTopics.size();
Topic* topic = availableTopics[index];
topic->studentId = studentId;
selections.push_back(*topic);
return topic;
}
// 查询选题结果
void querySelections() {
for (auto& selection : selections) {
cout << selection.studentId << " " << selection.id << endl;
}
}
int main() {
readStudents();
readTopics();
while (true) {
cout << "请输入学生学号(输入 q 退出):";
string studentId;
cin >> studentId;
if (studentId == "q") {
break;
}
bool found = false;
for (auto& student : students) {
if (student.id == studentId) {
found = true;
Topic* topic = selectTopic(studentId);
if (topic == nullptr) {
cout << "所有题目都已被选完!" << endl;
} else {
cout << "您的题目是:" << topic->title << endl;
}
break;
}
}
if (!found) {
cout << "学生不存在!" << endl;
}
}
cout << "选题结果如下:" << endl;
querySelections();
return 0;
}
```
注意:本代码仅供参考,实际使用时需要根据具体需求进行修改和完善。
相关推荐
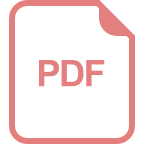
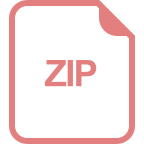














