第一题:输入10个整数,将其中最小的数与第1个数对换,把最大的数与最后一个数对换。编写3个子函数:(1)输入10个数;(2)进行处理;(3)输出10个数。 第二题: (1)编写一个子函数实现月份输出 (2)再编写一个子函数实现月份按字符串顺序排列,并将排序结果进行输出
时间: 2024-02-05 08:11:26 浏览: 63
第一题的Python代码实现:
```python
def input_nums():
nums = []
for i in range(10):
num = int(input("请输入第{}个整数:".format(i+1)))
nums.append(num)
return nums
def process_nums(nums):
min_index = nums.index(min(nums))
nums[0], nums[min_index] = nums[min_index], nums[0]
max_index = nums.index(max(nums))
nums[-1], nums[max_index] = nums[max_index], nums[-1]
return nums
def output_nums(nums):
for num in nums:
print(num, end=" ")
nums = input_nums()
nums_processed = process_nums(nums)
output_nums(nums_processed)
```
第二题的Python代码实现:
```python
# 子函数1:实现月份输出
def print_month(month):
month_dict = {
1: "一月",
2: "二月",
3: "三月",
4: "四月",
5: "五月",
6: "六月",
7: "七月",
8: "八月",
9: "九月",
10: "十月",
11: "十一月",
12: "十二月"
}
print(month_dict.get(month))
# 子函数2:实现月份按字符串顺序排列
def sort_months(months):
month_dict = {
"一月": 1,
"二月": 2,
"三月": 3,
"四月": 4,
"五月": 5,
"六月": 6,
"七月": 7,
"八月": 8,
"九月": 9,
"十月": 10,
"十一月": 11,
"十二月": 12
}
sorted_months = sorted(months, key=lambda x: month_dict[x])
return sorted_months
# 测试子函数1
print_month(3) # 输出:三月
# 测试子函数2
months = ["十月", "一月", "七月", "六月", "三月"]
sorted_months = sort_months(months)
print(sorted_months) # 输出:['一月', '三月', '六月', '七月', '十月']
```
阅读全文
相关推荐
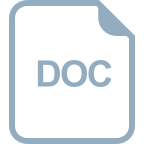
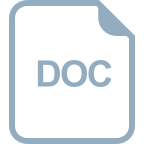
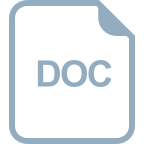















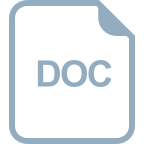