使用wpf开发一个动态datagrid,其中有一列是下拉框,采用模板列的方式,且需要将下拉框的选择的数据双向绑定到datagrid的数据源中,包含数据源的完整示例
时间: 2024-02-26 07:59:00 浏览: 76
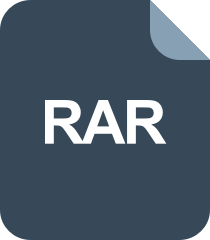
wpf下的DataGrid实例

好的,下面提供一个包含数据源的完整示例:
首先,我们需要创建一个 ViewModel 类,用于绑定到 DataGrid 的 ItemsSource 属性上。这个 ViewModel 类中需要包含两个属性:一个是 DataGrid 中需要显示的数据,另一个是下拉框的选项列表。
```
public class ViewModel : INotifyPropertyChanged
{
private ObservableCollection<DataItem> _items;
public ObservableCollection<DataItem> Items
{
get { return _items; }
set
{
_items = value;
OnPropertyChanged(nameof(Items));
}
}
private List<string> _comboBoxItems;
public List<string> ComboBoxItems
{
get { return _comboBoxItems; }
set
{
_comboBoxItems = value;
OnPropertyChanged(nameof(ComboBoxItems));
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
public class DataItem : INotifyPropertyChanged
{
private string _name;
public string Name
{
get { return _name; }
set
{
_name = value;
OnPropertyChanged(nameof(Name));
}
}
private string _comboBoxSelectedValue;
public string ComboBoxSelectedValue
{
get { return _comboBoxSelectedValue; }
set
{
_comboBoxSelectedValue = value;
OnPropertyChanged(nameof(ComboBoxSelectedValue));
}
}
public event PropertyChangedEventHandler PropertyChanged;
private void OnPropertyChanged(string propertyName)
{
PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName));
}
}
```
然后,在 MainWindow.xaml.cs 文件中,我们需要创建一个 ViewModel 实例,并将其绑定到 MainWindow 的 DataContext 上。在 ViewModel 中,我们需要初始化 Items 和 ComboBoxItems 属性。
```
public partial class MainWindow : Window
{
private ViewModel _viewModel;
public MainWindow()
{
InitializeComponent();
_viewModel = new ViewModel();
DataContext = _viewModel;
// 初始化 Items 属性
_viewModel.Items = new ObservableCollection<DataItem>
{
new DataItem { Name = "Item1", ComboBoxSelectedValue = "Option1" },
new DataItem { Name = "Item2", ComboBoxSelectedValue = "Option2" },
new DataItem { Name = "Item3", ComboBoxSelectedValue = "Option3" },
};
// 初始化 ComboBoxItems 属性
_viewModel.ComboBoxItems = new List<string>
{
"Option1",
"Option2",
"Option3",
};
}
}
```
最后,在 MainWindow.xaml 文件中,我们需要创建一个 DataGrid 控件,并添加一个模板列,用于显示下拉框。在模板列中,我们需要将 ComboBox 控件的 ItemsSource 属性绑定到 ComboBoxItems 属性上,将 ComboBox 控件的 SelectedItem 属性绑定到 ComboBoxSelectedValue 属性上。
```
<Window x:Class="WpfApp1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="clr-namespace:WpfApp1"
Title="MainWindow" Height="450" Width="800">
<Grid>
<DataGrid ItemsSource="{Binding Items}" AutoGenerateColumns="False">
<DataGrid.Columns>
<DataGridTextColumn Header="名称" Binding="{Binding Name}" />
<DataGridTemplateColumn Header="下拉框列">
<DataGridTemplateColumn.CellTemplate>
<DataTemplate>
<ComboBox ItemsSource="{Binding DataContext.ComboBoxItems, RelativeSource={RelativeSource AncestorType=DataGrid}}"
SelectedItem="{Binding ComboBoxSelectedValue, Mode=TwoWay}" />
</DataTemplate>
</DataGridTemplateColumn.CellTemplate>
</DataGridTemplateColumn>
</DataGrid.Columns>
</DataGrid>
</Grid>
</Window>
```
需要注意的是,ComboBox 控件的 ItemsSource 属性需要通过 RelativeSource 绑定到 DataGrid 的 DataContext 上。
希望这个完整示例能够帮到你!
阅读全文
相关推荐




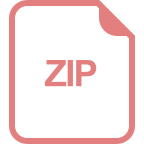
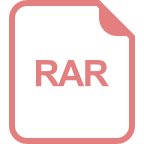
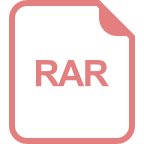
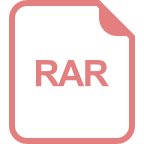
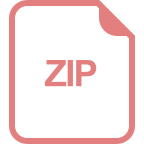
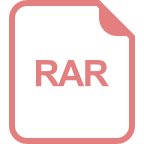
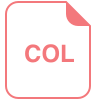



