qt MQTT 连接阿里云
时间: 2023-07-25 21:39:46 浏览: 238
连接阿里云MQTT需要以下步骤:
1. 在阿里云控制台创建一个MQTT实例,并获取实例的连接信息,包括Broker地址、端口号、用户名和密码等。
2. 在Qt项目中使用paho.mqtt.c库或MQTT-C++库实现MQTT连接,需要在项目中添加对应的头文件和库文件。
3. 在代码中设置MQTT连接参数,包括Broker地址、端口号、用户名和密码等。
4. 实现MQTT回调函数,处理MQTT消息的接收和发送。
以下是一个使用MQTT-C++库连接阿里云MQTT的示例代码:
```cpp
#include <iostream>
#include <mqtt/async_client.h>
const std::string SERVER_ADDRESS("tcp://<your-broker-address>:<your-broker-port>");
const std::string CLIENT_ID("<your-client-id>");
const std::string TOPIC("<your-topic>");
const int QOS = 1;
class mqtt_callback : public virtual mqtt::callback {
public:
virtual void connection_lost(const std::string& cause) {
std::cout << "Connection lost: " << cause << std::endl;
}
virtual void message_arrived(mqtt::const_message_ptr msg) {
std::cout << "Message arrived: " << msg->get_payload_str() << std::endl;
}
virtual void delivery_complete(mqtt::delivery_token_ptr token) {
std::cout << "Delivery complete for token: " << token->get_message_id() << std::endl;
}
};
int main(int argc, char* argv[]) {
mqtt::async_client client(SERVER_ADDRESS, CLIENT_ID);
mqtt::connect_options conn_opts;
conn_opts.set_keep_alive_interval(20);
conn_opts.set_clean_session(true);
conn_opts.set_user_name("<your-username>");
conn_opts.set_password("<your-password>");
mqtt_callback cb;
client.set_callback(cb);
try {
mqtt::token_ptr conntok = client.connect(conn_opts);
conntok->wait();
mqtt::token_ptr subtok = client.subscribe(TOPIC, QOS);
subtok->wait();
while (true) {
mqtt::message_ptr pubmsg = mqtt::make_message(TOPIC, "Hello, world!");
pubmsg->set_qos(QOS);
mqtt::token_ptr pubtok = client.publish(pubmsg);
pubtok->wait();
}
mqtt::token_ptr unsubtok = client.unsubscribe(TOPIC);
unsubtok->wait();
mqtt::token_ptr disctok = client.disconnect();
disctok->wait();
}
catch (const mqtt::exception& exc) {
std::cerr << "MQTT Exception: " << exc.what() << std::endl;
return 1;
}
return 0;
}
```
在这个例子中,我们使用了mqtt::async_client类来创建MQTT连接,设置连接参数并订阅一个主题。在主循环中,我们发送一个消息并等待回复。当程序退出时,我们取消订阅并断开连接。
注意:在实际使用中,需要将<your-broker-address>、<your-broker-port>、<your-client-id>、<your-username>和<your-password>替换为阿里云MQTT实例的具体信息。
相关推荐
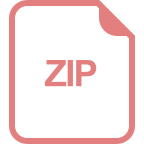
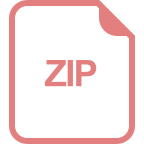
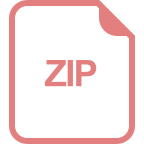














