python将圆环图和二维列表导入到word
时间: 2023-11-22 07:52:07 浏览: 33
要将圆环图和二维列表导入到Word中,可以使用Python的docx模块来实现。首先,需要安装docx模块:
```
pip install python-docx
```
接下来,可以使用以下代码创建一个新的Word文档,并向其中添加圆环图和二维列表:
```python
from docx import Document
from docx.shared import Inches, RGBColor
from docx.enum.text import WD_ALIGN_PARAGRAPH
from docx.oxml.ns import qn
from docx.chart import (
Chart,
DoughnutChart,
Series,
DataPoint,
)
from docx.table import Table, TableRow, TableCell
# 创建一个新的word文档
document = Document()
# 添加一个标题
document.add_heading('示例文档', level=0)
# 添加一个圆环图
chart_data = {
'Python': 60,
'Java': 30,
'C++': 10,
}
chart = DoughnutChart()
plot = chart.plots[0]
plot.height = Inches(2.0)
plot.width = Inches(4.0)
chart.title = '编程语言使用情况'
chart.style = 10
chart.legend = None
chart_data_labels = chart.plots[0].data_labels
chart_data_labels.number_format = '0"%"'
for key, value in chart_data.items():
series = Series([DataPoint(value=value)], title=key)
chart.series.append(series)
document.add_paragraph().add_run().add_chart(chart)
# 添加一个二维列表
data = [
['姓名', '年龄', '性别'],
['张三', '20', '男'],
['李四', '25', '女'],
['王五', '30', '男'],
]
table = document.add_table(rows=len(data), cols=len(data[0]))
table.style = 'Table Grid'
for i, row_data in enumerate(data):
row = table.rows[i]
for j, cell_data in enumerate(row_data):
cell = row.cells[j]
cell.text = cell_data
# 保存文档
document.save('示例文档.docx')
```
这段代码会创建一个新的Word文档,并向其中添加一个圆环图和一个二维列表。在添加圆环图时,使用了docx模块提供的Chart类来创建图表,并使用add_chart()方法将其添加到文档中。在添加二维列表时,使用了docx模块提供的Table类来创建表格,并使用add_table()方法将其添加到文档中。最后,使用save()方法保存文档。
注意,圆环图和二维列表的数据都需要在代码中预先定义,这里只是给出了示例数据。如果需要从外部文件读取数据,可以使用Python的文件操作功能来读取数据,并将其转换为适合文档中的格式。
相关推荐
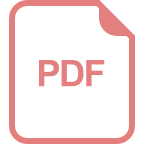
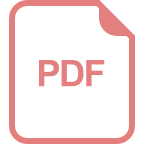
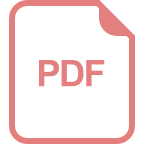
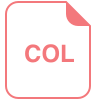
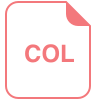
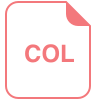
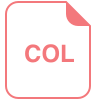
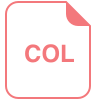









