复矩阵即元素为复效类型的矩阵,为简化要求,本例中只考虑矩阵元素类型均为复致类型的情况, 1.从面向对象的角度,实现复数矩阵,定义矩阵类及复数类,创建相关复矩阵。 2.复数类ComplexNurm的声明在文件ComplexNum.h中完成,并在ComplexNum.cpp文件 完成相关困数的定义。3.矩阵类Matrix的声明在文件Matrix.h中完成,并在Matix cpp文件完成相关函数的定义。 4.相关调用示例已在main图数中明确,请梦照调用完成相关困数定义。 5.要求程序可以正常运行,能在控制台输出要求的相关结果。 main通数所在源文件test.cppint main() { //创建4个复数对象 ComplexNum c1(2, 3), c2(4, 5), c3(6.7), c4(8, 9) //为简化代码处理,构建复数数组 ComplexNum sum = c1 + c2; cout << sum << endl; cout << "以下为复数矩阵" << endl; //构建2*2的复数矩阵 Matrix m(2, 2); for (int i = 0; i < 2 * 2; i++) { m.p[i] = c[i]; } //控制台打印矩阵 m.print(); return 0; }
时间: 2023-06-15 21:03:28 浏览: 80
以下是复数类ComplexNum的声明和定义:
ComplexNum.h
```
#pragma once
class ComplexNum
{
public:
ComplexNum(); // 默认构造函数
ComplexNum(double r, double i = 0); // 构造函数
ComplexNum(const ComplexNum& other); // 拷贝构造函数
~ComplexNum(); // 析构函数
ComplexNum operator+(const ComplexNum& other) const; // 加法运算
ComplexNum operator-(const ComplexNum& other) const; // 减法运算
ComplexNum operator*(const ComplexNum& other) const; // 乘法运算
ComplexNum operator/(const ComplexNum& other) const; // 除法运算
friend std::ostream& operator<<(std::ostream& os, const ComplexNum& c); // 输出运算符重载
private:
double real; // 实部
double imag; // 虚部
};
```
ComplexNum.cpp
```
#include "ComplexNum.h"
ComplexNum::ComplexNum() : real(0), imag(0)
{
}
ComplexNum::ComplexNum(double r, double i) : real(r), imag(i)
{
}
ComplexNum::ComplexNum(const ComplexNum& other) : real(other.real), imag(other.imag)
{
}
ComplexNum::~ComplexNum()
{
}
ComplexNum ComplexNum::operator+(const ComplexNum& other) const
{
return ComplexNum(real + other.real, imag + other.imag);
}
ComplexNum ComplexNum::operator-(const ComplexNum& other) const
{
return ComplexNum(real - other.real, imag - other.imag);
}
ComplexNum ComplexNum::operator*(const ComplexNum& other) const
{
double r = real * other.real - imag * other.imag;
double i = real * other.imag + imag * other.real;
return ComplexNum(r, i);
}
ComplexNum ComplexNum::operator/(const ComplexNum& other) const
{
double r = (real * other.real + imag * other.imag) / (other.real * other.real + other.imag * other.imag);
double i = (imag * other.real - real * other.imag) / (other.real * other.real + other.imag * other.imag);
return ComplexNum(r, i);
}
std::ostream& operator<<(std::ostream& os, const ComplexNum& c)
{
if (c.imag >= 0)
{
os << c.real << "+" << c.imag << "i";
}
else
{
os << c.real << c.imag << "i";
}
return os;
}
```
以下是矩阵类Matrix的声明和定义:
Matrix.h
```
#pragma once
#include "ComplexNum.h"
class Matrix
{
public:
Matrix(int rows, int cols); // 构造函数
Matrix(const Matrix& other); // 拷贝构造函数
~Matrix(); // 析构函数
ComplexNum& operator()(int i, int j); // 重载括号运算符
const ComplexNum& operator()(int i, int j) const; // 重载括号运算符
Matrix operator+(const Matrix& other) const; // 加法运算
Matrix operator-(const Matrix& other) const; // 减法运算
Matrix operator*(const Matrix& other) const; // 乘法运算
void print() const; // 打印矩阵
private:
int rows; // 行数
int cols; // 列数
ComplexNum* p; // 指向复数数组的指针
};
```
Matrix.cpp
```
#include "Matrix.h"
#include <iostream>
Matrix::Matrix(int rows, int cols) : rows(rows), cols(cols)
{
p = new ComplexNum[rows * cols];
}
Matrix::Matrix(const Matrix& other) : rows(other.rows), cols(other.cols)
{
p = new ComplexNum[rows * cols];
for (int i = 0; i < rows * cols; i++)
{
p[i] = other.p[i];
}
}
Matrix::~Matrix()
{
delete[] p;
}
ComplexNum& Matrix::operator()(int i, int j)
{
return p[i * cols + j];
}
const ComplexNum& Matrix::operator()(int i, int j) const
{
return p[i * cols + j];
}
Matrix Matrix::operator+(const Matrix& other) const
{
if (rows != other.rows || cols != other.cols)
{
std::cerr << "矩阵加法运算错误:矩阵大小不一致" << std::endl;
exit(1);
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
result(i, j) = (*this)(i, j) + other(i, j);
}
}
return result;
}
Matrix Matrix::operator-(const Matrix& other) const
{
if (rows != other.rows || cols != other.cols)
{
std::cerr << "矩阵减法运算错误:矩阵大小不一致" << std::endl;
exit(1);
}
Matrix result(rows, cols);
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
result(i, j) = (*this)(i, j) - other(i, j);
}
}
return result;
}
Matrix Matrix::operator*(const Matrix& other) const
{
if (cols != other.rows)
{
std::cerr << "矩阵乘法运算错误:矩阵大小不匹配" << std::endl;
exit(1);
}
Matrix result(rows, other.cols);
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < other.cols; j++)
{
ComplexNum sum(0, 0);
for (int k = 0; k < cols; k++)
{
sum = sum + (*this)(i, k) * other(k, j);
}
result(i, j) = sum;
}
}
return result;
}
void Matrix::print() const
{
for (int i = 0; i < rows; i++)
{
for (int j = 0; j < cols; j++)
{
std::cout << (*this)(i, j) << " ";
}
std::cout << std::endl;
}
}
```
最后在test.cpp中调用:
```
#include <iostream>
#include "Matrix.h"
int main()
{
// 创建4个复数对象
ComplexNum c1(2, 3), c2(4, 5), c3(6.7), c4(8, 9);
// 为简化代码处理,构建复数数组
ComplexNum c[4] = { c1, c2, c3, c4 };
// 构建2*2的复数矩阵
Matrix m(2, 2);
for (int i = 0; i < 2 * 2; i++)
{
m(i / 2, i % 2) = c[i];
}
// 控制台打印矩阵
std::cout << "以下为复数矩阵:" << std::endl;
m.print();
return 0;
}
```
相关推荐
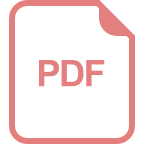
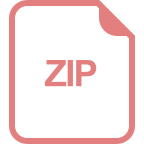
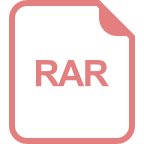










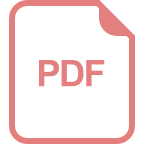
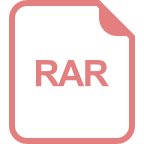
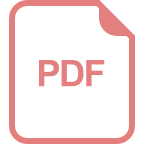
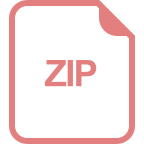
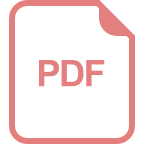