yolov3-tiny人脸检测
时间: 2023-07-04 16:09:45 浏览: 87
Yolov3-tiny是一种非常流行的目标检测算法,可以用于人脸检测。如果你想使用yolov3-tiny进行人脸检测,可以按照以下步骤:
1. 下载预训练的yolov3-tiny权重文件。
2. 安装OpenCV和Python。
3. 编写Python脚本,读取图像并使用yolov3-tiny进行人脸检测。
4. 显示检测结果。
以下是一个简单的Python脚本示例,用于使用yolov3-tiny进行人脸检测:
```python
import cv2
# 加载网络和权重文件
net = cv2.dnn.readNet("yolov3-tiny.weights", "yolov3-tiny.cfg")
# 加载图像
image = cv2.imread("test.jpg")
# 获取图像的高度和宽度
(h, w) = image.shape[:2]
# 设置参数
confidence_threshold = 0.5
nms_threshold = 0.4
# 构建输入图像
blob = cv2.dnn.blobFromImage(image, 1/255.0, (416, 416), swapRB=True, crop=False)
# 设置网络的输入
net.setInput(blob)
# 运行网络
outputs = net.forward(net.getUnconnectedOutLayersNames())
# 针对每个输出进行处理
for output in outputs:
for detection in output:
scores = detection[5:]
classId = np.argmax(scores)
confidence = scores[classId]
# 如果置信度大于阈值,则进行处理
if confidence > confidence_threshold:
# 计算边界框的位置
box = detection[0:4] * np.array([w, h, w, h])
(centerX, centerY, width, height) = box.astype("int")
# 计算边界框的左上角坐标
x = int(centerX - (width / 2))
y = int(centerY - (height / 2))
# 添加边界框和置信度
boxes.append([x, y, int(width), int(height)])
confidences.append(float(confidence))
classIDs.append(classId)
# 应用非最大抑制
indices = cv2.dnn.NMSBoxes(boxes, confidences, confidence_threshold, nms_threshold)
# 循环遍历保留下来的边界框
for i in indices:
i = i[0]
box = boxes[i]
(x, y, w, h) = box
# 绘制边界框和置信度
cv2.rectangle(image, (x, y), (x + w, y + h), (0, 255, 0), 2)
text = "{}: {:.4f}".format("face", confidences[i])
cv2.putText(image, text, (x, y - 5), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
# 显示结果
cv2.imshow("Output", image)
cv2.waitKey(0)
```
请注意,此代码仅是示例,可能需要根据您的需求进行修改。
相关推荐
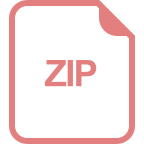
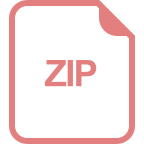














