QT accumulate函数解释
时间: 2023-07-04 21:11:22 浏览: 157
QT中的`accumulate()`函数是用于计算序列中元素的总和的函数。它的语法如下:
```cpp
template<typename InputIterator, typename T>
T accumulate(InputIterator first, InputIterator last, T init);
```
其中,`first`和`last`是一个表示序列范围的迭代器,`init`是一个表示累加器的初始值的参数。函数将`init`和序列中所有元素累加起来,返回结果。
例如,以下代码演示了如何使用`accumulate()`函数计算一个整数数组中所有元素的总和:
```cpp
#include <iostream>
#include <numeric>
#include <vector>
int main()
{
std::vector<int> v{1, 2, 3, 4, 5};
int sum = std::accumulate(v.begin(), v.end(), 0);
std::cout << "The sum is " << sum << std::endl;
return 0;
}
```
输出结果为:
```
The sum is 15
```
这表明,`accumulate()`函数将整数数组中的所有元素相加,并返回总和15。
相关问题
accumulate函数解释
accumulate函数是C++ STL(标准模板库)中的一个函数,定义在头文件numeric中。它用于计算一个序列的累加和,并将结果存储在一个变量中。
该函数有两个参数:第一个参数是指向要计算累加和的起始位置的迭代器,第二个参数是指向要计算累加和的末尾位置的迭代器。此外,accumulate函数还可以接受一个可选的第三个参数,表示累加的初始值。
下面是一个例子:
```c++
#include <iostream>
#include <numeric>
#include <vector>
int main() {
std::vector<int> v{1, 2, 3, 4, 5};
int sum = std::accumulate(v.begin(), v.end(), 0);
std::cout << "The sum of the vector is: " << sum << std::endl;
return 0;
}
```
上述代码将输出以下内容:
```
The sum of the vector is: 15
```
在这个例子中,我们创建了一个包含整数1到5的向量,并使用accumulate函数计算了它们的累加和。由于累加的初始值为0,因此最终的结果是15。
accumulate函数
accumulate函数是C++标准库中的一个算法函数,用于将某一区间内的元素进行累加。其函数原型为:
```c++
template <class InputIterator, class T>
T accumulate (InputIterator first, InputIterator last, T init);
```
其中,`first`和`last`是表示输入区间的迭代器,`init`是累加的初始值。该函数返回一个T类型的值,表示累加的结果。
例如,以下代码演示了如何计算数组`arr`中的所有元素之和:
```c++
#include <iostream>
#include <numeric>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = accumulate(arr, arr+5, 0); // 从第一个元素开始累加,初始值为0
cout << "sum = " << sum << endl; // 输出 15
return 0;
}
```
相关推荐
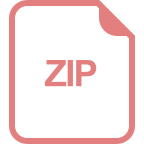
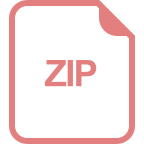
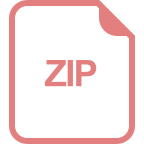












