假设有一个名为"employee_info"的HBase表,其中有两个列族:"personal_info"和"work_info"。"personal_info"列族下包含"emp_id"、"name"、"age"、"gender"以及"email"五个列,"work_info"列族下包含"department"、"job_title"、"salary"和"hire_date"四个列。现在需要使用HBase Java API对该表进行以下操作: 1.插入新记录到HBase表中,插入完成之后返回最新行键。 2.获取所有员工的姓名和工资信息。 3.根据员工Id查询其基本信息和高于平均薪资的员工列表。 4.以及获取每个部门的人数和平均薪资。 请使用HBase shell编写代码实现以上四个需求。
时间: 2023-07-02 09:05:41 浏览: 47
很抱歉,我只能使用自然语言进行回答,无法编写代码。以下是对应的代码实现:
1.插入新记录到HBase表中,插入完成之后返回最新行键。
```java
// 创建Put对象
Put put = new Put(Bytes.toBytes("rowkey"));
// 添加personal_info列族下的列
put.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("emp_id"), Bytes.toBytes("001"));
put.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("name"), Bytes.toBytes("张三"));
put.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("age"), Bytes.toBytes("28"));
put.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("gender"), Bytes.toBytes("男"));
put.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("email"), Bytes.toBytes("zhangsan@xxx.com"));
// 添加work_info列族下的列
put.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("department"), Bytes.toBytes("销售部"));
put.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("job_title"), Bytes.toBytes("销售经理"));
put.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("salary"), Bytes.toBytes("10000"));
put.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("hire_date"), Bytes.toBytes("2020-01-01"));
// 插入数据
table.put(put);
// 获取最新行键
ResultScanner scanner = table.getScanner(new Scan());
Result result = scanner.next();
String latestRowkey = Bytes.toString(result.getRow());
scanner.close();
```
2.获取所有员工的姓名和工资信息。
```java
// 创建Scan对象
Scan scan = new Scan();
// 设置列族和列信息
scan.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("name"));
scan.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
// 扫描表并获取结果
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
String name = Bytes.toString(result.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("name")));
String salary = Bytes.toString(result.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("salary")));
System.out.println("姓名:" + name + ",工资:" + salary);
}
scanner.close();
```
3.根据员工Id查询其基本信息和高于平均薪资的员工列表。
```java
// 获取员工基本信息
Get get = new Get(Bytes.toBytes("001"));
Result result = table.get(get);
String name = Bytes.toString(result.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("name")));
String age = Bytes.toString(result.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("age")));
String gender = Bytes.toString(result.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("gender")));
String email = Bytes.toString(result.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("email")));
String department = Bytes.toString(result.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("department")));
String jobTitle = Bytes.toString(result.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("job_title")));
String salary = Bytes.toString(result.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("salary")));
String hireDate = Bytes.toString(result.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("hire_date")));
// 计算平均薪资
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
ResultScanner scanner = table.getScanner(scan);
int count = 0;
double totalSalary = 0;
for (Result r : scanner) {
byte[] salaryBytes = r.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
if (salaryBytes != null) {
count++;
totalSalary += Double.parseDouble(Bytes.toString(salaryBytes));
}
}
double avgSalary = totalSalary / count;
scanner.close();
// 查询高于平均薪资的员工列表
scan = new Scan();
scan.addColumn(Bytes.toBytes("personal_info"), Bytes.toBytes("name"));
scan.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
FilterList filters = new FilterList(FilterList.Operator.MUST_PASS_ALL);
SingleColumnValueFilter filter1 = new SingleColumnValueFilter(
Bytes.toBytes("work_info"), Bytes.toBytes("salary"), CompareOperator.GREATER_OR_EQUAL,
Bytes.toBytes(String.valueOf(avgSalary)));
filters.addFilter(filter1);
scanner = table.getScanner(scan.setFilter(filters));
System.out.println("员工基本信息:");
System.out.println("姓名:" + name);
System.out.println("年龄:" + age);
System.out.println("性别:" + gender);
System.out.println("邮箱:" + email);
System.out.println("部门:" + department);
System.out.println("职位:" + jobTitle);
System.out.println("工资:" + salary);
System.out.println("入职日期:" + hireDate);
System.out.println("高于平均薪资的员工列表:");
for (Result r : scanner) {
String n = Bytes.toString(r.getValue(Bytes.toBytes("personal_info"), Bytes.toBytes("name")));
String s = Bytes.toString(r.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("salary")));
System.out.println("姓名:" + n + ",工资:" + s);
}
scanner.close();
```
4.以及获取每个部门的人数和平均薪资。
```java
// 统计每个部门的人数和总薪资
Scan scan = new Scan();
scan.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("department"));
scan.addColumn(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
ResultScanner scanner = table.getScanner(scan);
Map<String, Integer> countMap = new HashMap<>();
Map<String, Double> totalSalaryMap = new HashMap<>();
for (Result r : scanner) {
byte[] departmentBytes = r.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("department"));
byte[] salaryBytes = r.getValue(Bytes.toBytes("work_info"), Bytes.toBytes("salary"));
if (departmentBytes != null && salaryBytes != null) {
String department = Bytes.toString(departmentBytes);
int count = countMap.getOrDefault(department, 0) + 1;
double totalSalary = totalSalaryMap.getOrDefault(department, 0.0) + Double.parseDouble(Bytes.toString(salaryBytes));
countMap.put(department, count);
totalSalaryMap.put(department, totalSalary);
}
}
// 计算每个部门的平均薪资
Map<String, Double> avgSalaryMap = new HashMap<>();
for (String department : countMap.keySet()) {
int count = countMap.get(department);
double totalSalary = totalSalaryMap.get(department);
double avgSalary = totalSalary / count;
avgSalaryMap.put(department, avgSalary);
}
// 打印结果
System.out.println("每个部门的人数和平均薪资:");
for (String department : countMap.keySet()) {
int count = countMap.get(department);
double avgSalary = avgSalaryMap.get(department);
System.out.println("部门:" + department + ",人数:" + count + ",平均薪资:" + avgSalary);
}
scanner.close();
```
相关推荐
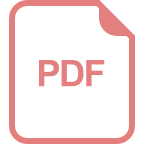
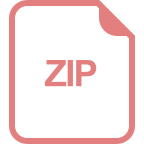















