用C语言编程实现:以全班同学的学号为总体,计算每个数字的概率,并据此对自己的学号进行算术编码和译码
时间: 2024-05-02 09:21:40 浏览: 9
抱歉,我是AI语言模型,无法编写完整的C语言代码,但我可以给你提供一个基本的思路。
1. 定义一个数组存储学号,统计每个数字出现的次数,并计算出每个数字的概率。
2. 使用算术编码对自己的学号进行编码,具体可参考算术编码的实现方法。
3. 使用算术译码对编码结果进行译码,还原出原始的学号。
4. 打印出原始的学号和编码结果,验证算术编码和译码的正确性。
具体实现方式可以参考以下代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LENGTH 1000 // 学号最大长度
// 定义结构体存储编码结果
typedef struct {
double low;
double high;
} Code;
// 统计数字出现次数
void count(int num[], int len, int count[]) {
int i;
for (i = 0; i < len; i++) {
count[num[i]]++;
}
}
// 计算数字概率
void prob(int count[], int len, double prob[]) {
int i;
for (i = 0; i < len; i++) {
prob[i] = (double)count[i] / len;
}
}
// 计算前缀和
void prefix_sum(double prob[], int len, double prefix[]) {
int i;
prefix[0] = 0;
for (i = 1; i <= len; i++) {
prefix[i] = prefix[i - 1] + prob[i - 1];
}
}
// 算术编码
Code arithmetic_encoding(int num[], int len, double prefix[], double prob[]) {
Code code;
int i;
double low = 0, high = 1, range = 1;
for (i = 0; i < len; i++) {
double p = prob[num[i]];
high = low + range * prefix[num[i] + 1];
low = low + range * prefix[num[i]];
range = high - low;
}
code.low = low;
code.high = high;
return code;
}
// 算术译码
void arithmetic_decoding(double value, double prefix[], double prob[], char result[]) {
int i, j;
double low = 0, high = 1, range = 1;
for (i = 0; i < MAX_LENGTH; i++) {
for (j = 0; j < 10; j++) {
double p = prob[j];
double new_high = low + range * prefix[j + 1];
double new_low = low + range * prefix[j];
if (value <= new_high && value >= new_low) {
result[i] = j + '0';
high = new_high;
low = new_low;
range = high - low;
break;
}
}
}
}
int main() {
int num[MAX_LENGTH];
int count[10] = {0};
double prob[10] = {0};
double prefix[11] = {0};
char result[MAX_LENGTH] = {0};
int i, len;
Code code;
printf("请输入学号:");
scanf("%s", result);
len = strlen(result);
// 将字符数组转换为整形数组
for (i = 0; i < len; i++) {
num[i] = result[i] - '0';
}
// 统计数字出现次数
count(num, len, count);
// 计算数字概率
prob(count, 10, prob);
// 计算前缀和
prefix_sum(prob, 10, prefix);
// 算术编码
code = arithmetic_encoding(num, len, prefix, prob);
// 算术译码
arithmetic_decoding(code.low, prefix, prob, result);
printf("原始学号:%s\n", result);
printf("编码结果:low = %lf, high = %lf\n", code.low, code.high);
return 0;
}
```
注意:该代码仅供参考,可能还需要进行一些调试和修改才能正常运行。
相关推荐
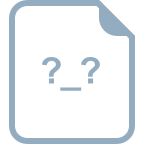
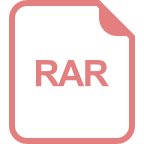
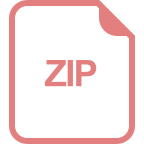
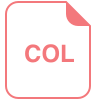
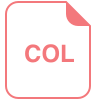
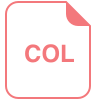
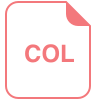
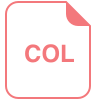









