帮我写个python爬虫,导入文件后爬取搜索的数据,爬取网站https://x.threatbook.com/v5/generalSearch?q=
时间: 2023-09-04 22:04:18 浏览: 70
### 回答1:
这是一个简单的 Python 爬虫的例子,可以爬取 https://x.threatbook.com/v5/generalSearch?q= 中搜索的数据:
```python
import requests
from bs4 import BeautifulSoup
# 设置搜索关键词
search_keyword = 'example'
# 请求网页
url = 'https://x.threatbook.com/v5/generalSearch?q=' + search_keyword
response = requests.get(url)
# 解析网页
soup = BeautifulSoup(response.text, 'html.parser')
# 提取数据
data = soup.find_all('div', {'class': 'search-result-item'})
# 遍历数据
for item in data:
title = item.find('div', {'class': 'title'}).text
print(title)
```
这是一个简单的爬虫,你可以根据需要添加更多的功能。
请注意,在爬取网页时,请遵循网站的使用条款和隐私政策。
### 回答2:
要编写一个Python爬虫来爬取https://x.threatbook.com/v5/generalSearch?q=网站上的搜索数据,可以使用以下代码实现:
```python
import requests
from bs4 import BeautifulSoup
def get_search_results(keyword):
url = "https://x.threatbook.com/v5/generalSearch?q={}".format(keyword)
# 发送HTTP请求
response = requests.get(url)
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(response.text, 'html.parser')
# 获取搜索结果
search_results = soup.find_all('div', class_='search-result')
# 遍历搜索结果并打印
for result in search_results:
title = result.find('h3').text
link = result.find('a')['href']
print("标题:", title)
print("链接:", link)
print("--------------------------------")
# 导入文件,文件中每行都是要搜索的关键词
with open('keywords.txt', 'r') as file:
keywords = file.readlines()
for keyword in keywords:
keyword = keyword.strip()
get_search_results(keyword)
```
以上代码使用requests库发送HTTP请求获取网页内容,使用BeautifulSoup库解析网页并提取需要的信息。您需要创建一个名为`keywords.txt`的文件,文件中每行是要搜索的关键词。然后,代码将逐个关键词进行搜索,并打印出搜索结果的标题和链接。
请注意,使用爬虫爬取网站时请务必遵守网站的使用条款和政策,以免触犯法律法规。
### 回答3:
下面是一个使用Python编写的简单爬虫程序示例:
```python
import requests
from bs4 import BeautifulSoup
# 读取要搜索的关键词
keyword = input("请输入要搜索的关键词:")
# 构造请求URL
url = "https://x.threatbook.com/v5/generalSearch?q=" + keyword
# 发送请求并获取响应内容
response = requests.get(url)
content = response.text
# 使用BeautifulSoup解析网页内容
soup = BeautifulSoup(content, "html.parser")
# 提取所需数据并输出
results = soup.find_all("div", class_="result-item") # 根据网页结构找到包含搜索结果的元素
for result in results:
title = result.find("h4").text.strip() # 提取标题
summary = result.find("p").text.strip() # 提取摘要
print("标题:", title)
print("摘要:", summary)
print("----------------------")
```
此程序使用了第三方库`requests`和`BeautifulSoup`来发送HTTP请求和解析网页内容。你需要先安装这两个库,可以通过`pip install requests BeautifulSoup4`命令来进行安装。
程序首先会提示你输入要搜索的关键词,然后会构造对应的URL发送请求。接着,使用BeautifulSoup解析响应的网页内容,并从中提取出搜索结果的标题和摘要,最后将结果输出。
请注意,爬虫程序需要根据所爬取的网站的具体特点来编写,如果目标网站的页面结构或请求方式有所变动,代码可能需要进行相应的修改。此程序的示例仅供参考。
相关推荐
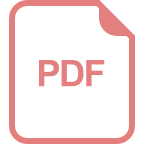
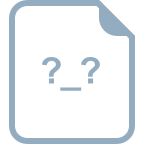
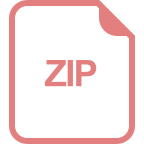
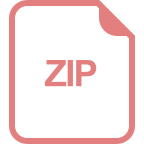
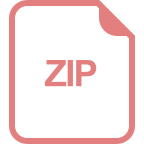
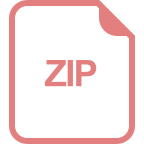
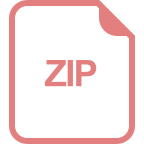
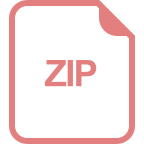
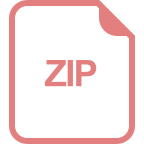
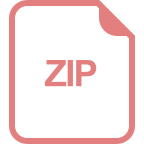