用python写一个会动的七彩蟒蛇
时间: 2024-05-13 22:20:01 浏览: 217
好的,以下是一个简单的Python代码,可以绘制出一个会动的七彩蟒蛇:
```python
import turtle
import random
colors = ["red", "orange", "yellow", "green", "blue", "purple"]
# 创建画布
wn = turtle.Screen()
wn.bgcolor("black")
wn.title("Rainbow Snake")
# 创建蛇头
head = turtle.Turtle()
head.shape("circle")
head.color(random.choice(colors))
head.penup()
head.goto(0, 0)
head.speed(0)
head.direction = "stop"
# 创建食物
food = turtle.Turtle()
food.shape("circle")
food.color("white")
food.penup()
food.goto(random.randint(-290, 290), random.randint(-290, 290))
# 移动函数
def move():
if head.direction == "up":
y = head.ycor()
head.sety(y + 20)
elif head.direction == "down":
y = head.ycor()
head.sety(y - 20)
elif head.direction == "right":
x = head.xcor()
head.setx(x + 20)
elif head.direction == "left":
x = head.xcor()
head.setx(x - 20)
# 转向函数
def go_up():
if head.direction != "down":
head.direction = "up"
head.color(random.choice(colors))
def go_down():
if head.direction != "up":
head.direction = "down"
head.color(random.choice(colors))
def go_right():
if head.direction != "left":
head.direction = "right"
head.color(random.choice(colors))
def go_left():
if head.direction != "right":
head.direction = "left"
head.color(random.choice(colors))
# 注册监听器
wn.listen()
wn.onkeypress(go_up, "Up")
wn.onkeypress(go_down, "Down")
wn.onkeypress(go_right, "Right")
wn.onkeypress(go_left, "Left")
while True:
wn.update()
# 碰到边界
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
head.goto(0, 0)
head.direction = "stop"
head.color(random.choice(colors))
# 吃到食物
if head.distance(food) < 20:
food.goto(random.randint(-290, 290), random.randint(-290, 290))
head.color(random.choice(colors))
# 移动蛇身
for index in range(len(turtles) - 1, 0, -1):
x = turtles[index - 1].xcor()
y = turtles[index - 1].ycor()
turtles[index].goto(x, y)
# 移动蛇头
move()
# 延迟
turtle.delay(0.1)
turtle.mainloop()
```
这个代码使用了Python的turtle库,在屏幕中绘制了一个七彩的蟒蛇。你可以使用上下左右键来控制蛇的移动方向,当蛇吃到食物时会变换颜色。
阅读全文
相关推荐
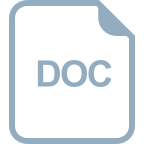
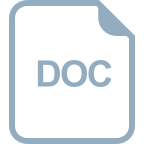
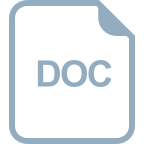






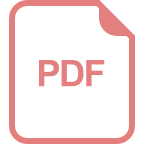
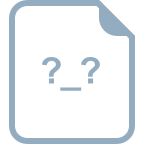

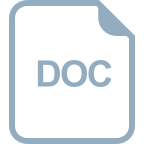
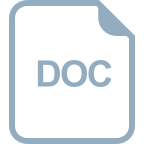