pyqt用QChart动态生成折线图
时间: 2023-10-25 18:25:09 浏览: 237
以下是一个简单的示例,将使用PyQt5和QChart生成动态折线图。
首先,我们需要导入必要的模块:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QSizePolicy, QWidget
from PyQt5.QtChart import QChart, QChartView, QLineSeries
from PyQt5.QtCore import Qt, QTime, QTimer
```
然后,我们创建一个主窗口类,并在其构造函数中创建一个QWidget实例,它将包含我们的QChartView。
```python
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
# Create a QWidget for the chart view
chart_widget = QWidget(self)
chart_widget.setGeometry(0, 0, 800, 600)
# Create a QChart and QChartView
chart = QChart()
chart.setTitle("Dynamic Line Chart")
chart.legend().hide()
chart_view = QChartView(chart, chart_widget)
chart_view.setSizePolicy(QSizePolicy.Expanding, QSizePolicy.Expanding)
chart_view.setRenderHint(QPainter.Antialiasing)
# Add the chart view to the widget
layout = QVBoxLayout(chart_widget)
layout.addWidget(chart_view)
# Initialize the line series
self.series = QLineSeries()
self.series.append(0, 0)
chart.addSeries(self.series)
# Set the X axis range
axis_x = chart.axisX()
axis_x.setRange(0, 10)
# Set the Y axis range
axis_y = chart.axisY()
axis_y.setRange(-1, 1)
# Create a timer to update the chart
self.timer = QTimer()
self.timer.timeout.connect(self.update_chart)
self.timer.start(1000)
def update_chart(self):
# Generate a random value
value = (2 * (random.random() - 0.5))
# Add the value to the series
count = self.series.count()
self.series.append(count, value)
# Remove the oldest value if the series is too long
if count > 10:
self.series.removePoints(0, count - 10)
```
在构造函数中,我们创建一个QWidget实例,它将包含我们的QChartView。我们还创建了一个QChart和QChartView,并将其添加到QWidget中。我们还初始化了一个QLineSeries,并将其添加到QChart中。我们设置了X和Y轴的范围,并创建了一个QTimer来每秒更新图表。
在update_chart函数中,我们生成一个随机值,并将其添加到QLineSeries中。如果QLineSeries太长,我们将删除最旧的值。
最后,我们在主函数中创建QApplication和MainWindow实例,并运行应用程序。
```python
if __name__ == "__main__":
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
```
阅读全文
相关推荐





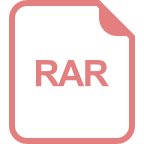



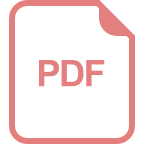
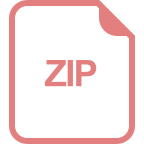
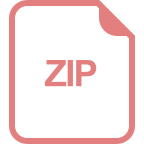
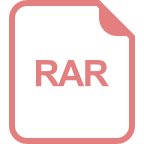
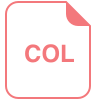
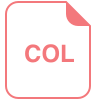
