用c++代码设计一个电子地图管理系统
时间: 2024-05-01 13:22:56 浏览: 83
好的,下面是一个简单的电子地图管理系统的设计:
```c++
#include <iostream>
#include <vector>
#include <string>
using namespace std;
class Location {
public:
string name; // 地点名称
double latitude; // 纬度
double longitude; // 经度
Location(string n, double la, double lo) : name(n), latitude(la), longitude(lo) {}
};
class Map {
public:
vector<Location> locations; // 存储所有地点信息
void addLocation(Location loc) {
locations.push_back(loc);
}
void printAllLocations() {
for (int i = 0; i < locations.size(); i++) {
cout << "地点名称:" << locations[i].name << endl;
cout << "经度:" << locations[i].longitude << endl;
cout << "纬度:" << locations[i].latitude << endl;
cout << endl;
}
}
void searchLocationByName(string name) {
bool found = false;
for (int i = 0; i < locations.size(); i++) {
if (locations[i].name == name) {
cout << "地点名称:" << locations[i].name << endl;
cout << "经度:" << locations[i].longitude << endl;
cout << "纬度:" << locations[i].latitude << endl;
found = true;
}
}
if (!found) {
cout << "未找到该地点!" << endl;
}
}
void searchLocationByCoordinate(double longitude, double latitude) {
bool found = false;
for (int i = 0; i < locations.size(); i++) {
if (locations[i].longitude == longitude && locations[i].latitude == latitude) {
cout << "地点名称:" << locations[i].name << endl;
cout << "经度:" << locations[i].longitude << endl;
cout << "纬度:" << locations[i].latitude << endl;
found = true;
}
}
if (!found) {
cout << "未找到该地点!" << endl;
}
}
};
int main() {
Map map;
Location loc1("清华大学", 39.9967, 116.3264);
Location loc2("北京大学", 39.9622, 116.3339);
Location loc3("中关村创业大街", 39.9848, 116.3074);
map.addLocation(loc1);
map.addLocation(loc2);
map.addLocation(loc3);
cout << "所有地点信息:" << endl;
map.printAllLocations();
cout << "按名称查找地点:" << endl;
map.searchLocationByName("清华大学");
cout << "按坐标查找地点:" << endl;
map.searchLocationByCoordinate(116.3339, 39.9622);
return 0;
}
```
该系统使用两个类来实现:`Location` 类和 `Map` 类。 `Location` 类表示一个地点,包含名称、纬度和经度三个属性。 `Map` 类表示整个电子地图系统,包含一个地点信息的向量,以及添加地点、打印所有地点、按名称或坐标查找地点等功能。
在 `main()` 函数中,我们创建了三个地点对象,并将它们添加到地图中。然后,我们通过调用 `Map` 类中的函数来打印所有地点信息,按名称查找地点和按坐标查找地点。
阅读全文
相关推荐
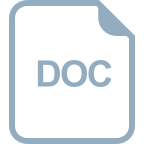
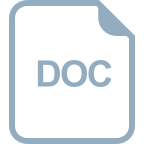
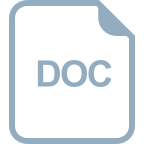
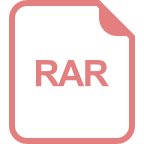
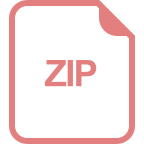
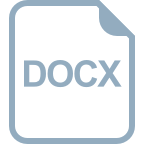
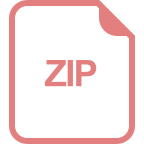
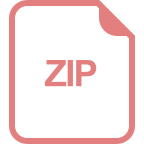
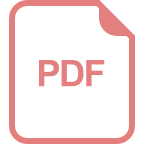
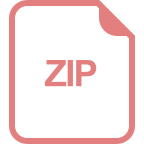
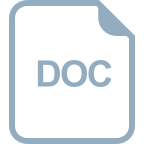
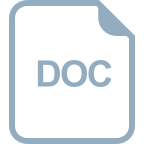
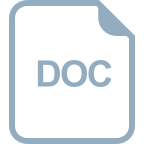
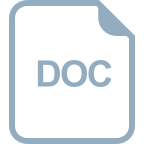
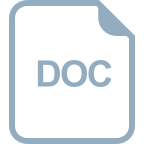
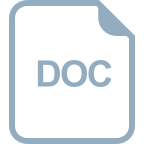

