哈夫曼树生成输入字符集的变长码 Java代码
时间: 2024-05-08 07:22:17 浏览: 45
以下是一个简单的 Java 代码实现,用于生成输入字符集的哈夫曼树和变长编码:
```java
import java.util.PriorityQueue;
class Node implements Comparable<Node> {
char ch;
int freq;
Node left, right;
Node(char ch, int freq, Node left, Node right) {
this.ch = ch;
this.freq = freq;
this.left = left;
this.right = right;
}
boolean isLeaf() {
return left == null && right == null;
}
@Override
public int compareTo(Node node) {
return freq - node.freq;
}
}
public class HuffmanTree {
private Node root;
public HuffmanTree(char[] chars, int[] freqs) {
PriorityQueue<Node> pq = new PriorityQueue<>();
for (int i = 0; i < chars.length; i++) {
pq.offer(new Node(chars[i], freqs[i], null, null));
}
while (pq.size() > 1) {
Node left = pq.poll();
Node right = pq.poll();
Node parent = new Node('\0', left.freq + right.freq, left, right);
pq.offer(parent);
}
root = pq.poll();
}
public void printCodes() {
printCodes(root, "");
}
private void printCodes(Node node, String code) {
if (node == null) {
return;
}
if (node.isLeaf()) {
System.out.println(node.ch + ": " + code);
}
printCodes(node.left, code + "0");
printCodes(node.right, code + "1");
}
}
```
在这个实现中,我们使用了一个优先队列来存储每个字符及其对应的频率。我们不断从队列中取出频率最小的两个节点,并将它们合并成一个新节点,直到队列中只剩下一个节点为止。这个节点就是哈夫曼树的根节点。
我们还实现了一个函数 `printCodes()`,用于打印每个字符对应的变长编码。这个函数使用了递归来遍历整个哈夫曼树,并将遇到的每个叶子节点的编码打印出来。
以下是一个使用示例:
```java
public static void main(String[] args) {
char[] chars = {'a', 'b', 'c', 'd', 'e', 'f'};
int[] freqs = {5, 9, 12, 13, 16, 45};
HuffmanTree tree = new HuffmanTree(chars, freqs);
tree.printCodes();
}
```
这个示例将会输出每个字符的变长编码:
```
f: 0
c: 100
d: 101
a: 1100
b: 1101
e: 111
```
阅读全文
相关推荐

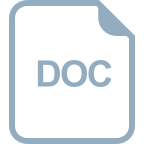
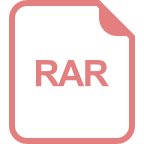
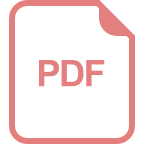
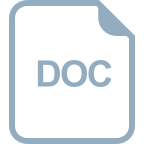
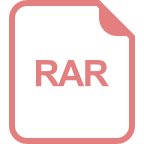
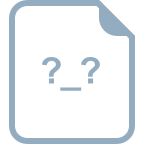
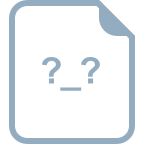
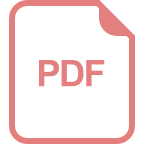
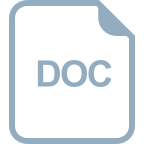
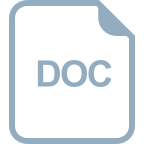
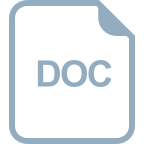
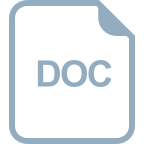
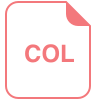
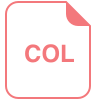