from imblearn.under_sampling import RandomUnderSampler
时间: 2024-02-23 14:01:24 浏览: 39
`imblearn` 是一个用于不平衡数据集处理的Python库,提供了多种处理不平衡数据集的方法,包括欠采样、过采样、组合采样等方法。`RandomUnderSampler` 是其中一种欠采样方法,它可以随机地从多数类中去除一些样本,从而达到平衡数据集的目的。使用方法如下:
```python
from imblearn.under_sampling import RandomUnderSampler
rus = RandomUnderSampler()
X_resampled, y_resampled = rus.fit_resample(X, y)
```
其中,`X` 表示特征矩阵,`y` 表示标签。`fit_resample` 方法可以对数据集进行欠采样,返回欠采样后的特征矩阵和标签。在 `RandomUnderSampler` 中,可以设置 `sampling_strategy` 参数来指定欠采样的比例,例如:
```python
rus = RandomUnderSampler(sampling_strategy=0.5)
```
这样将会从多数类中随机去除一半的样本。如果不指定 `sampling_strategy` 参数,则会默认将多数类的样本数减少到与少数类的样本数相等。
相关问题
from imblearn.over_sampling import SMOTE from imblearn.under_sampling import RandomUnderSampler from imblearn.pipeline import Pipeline # values to evaluate over_values = [0.3,0.4,0.5] under_values = [0.7,0.8,0.9] for o in over_values: for u in under_values: # define pipeline model = SVC() over = SMOTE(sampling_strategy=o) under = RandomUnderSampler(sampling_strategy=u) steps = [('over', over), ('under', under), ('model', model)] pipeline = Pipeline(steps=steps) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0) # 拟合模型 pipeline.fit(X_train, y_train) 显示The specified ratio required to remove samples from the minority class while trying to generate new samples. Please increase the ratio.该如何修改
在您的代码中,出现了警告信息"The specified ratio required to remove samples from the minority class while trying to generate new samples. Please increase the ratio." 这是因为在使用`RandomUnderSampler`进行欠采样时,您指定的`sampling_strategy`参数的值可能过小,导致无法满足要求的欠采样比例。
要解决这个问题,您可以尝试增加`sampling_strategy`参数的值。根据警告信息的建议,您可以尝试逐步增加欠采样比例,直到满足要求。
在您的代码中,尝试将欠采样比例逐步增加,例如:
```python
under_values = [0.7, 0.8, 0.9]
```
您可以根据具体情况调整这些值,并观察警告是否消失。如果警告仍然存在,您可能需要进一步增加欠采样比例,直到满足要求。
请注意,根据您的数据集和需求,可能需要根据实际情况调整欠采样比例的取值范围。
from imblearn.over_sampling import SMOTE from imblearn.under_sampling import RandomUnderSampler from imblearn.pipeline import Pipeline from sklearn.model_selection import cross_val_score # 定义管道 #欠采样和过采样的结合(使用pipeline) model = SVC() over = SMOTE(sampling_strategy=0.4) under = RandomUnderSampler(sampling_strategy=0.5) steps = [('o', over), ('u', under), ('model', model)] pipeline = Pipeline(steps=steps) # 评估效果 scores = cross_val_score(pipeline, X, y, scoring='roc_auc', cv=5, n_jobs=-1) score = np.mean(scores) print('ROC AUC score for the combined sampling method: %.3f' % score)该如何求该模型的accuracy,AUC ,precision,recall,f1 score ,Sensitivity ,Specificity 还要画出ROC曲线和混淆矩阵
要求该模型的accuracy,AUC,precision,recall,f1 score,Sensitivity,Specificity以及绘制ROC曲线和混淆矩阵,可以按照以下步骤进行:
1. 导入需要的库:
```python
import numpy as np
from sklearn.metrics import accuracy_score, roc_auc_score, precision_score, recall_score, f1_score, confusion_matrix, roc_curve
import matplotlib.pyplot as plt
```
2. 训练模型和预测结果:
```python
model.fit(X, y)
y_pred = model.predict(X)
```
3. 计算指标:
```python
accuracy = accuracy_score(y, y_pred)
auc = roc_auc_score(y, y_pred)
precision = precision_score(y, y_pred)
recall = recall_score(y, y_pred)
f1 = f1_score(y, y_pred)
```
4. 计算混淆矩阵:
```python
cm = confusion_matrix(y, y_pred)
```
5. 计算Sensitivity和Specificity:
```python
sensitivity = cm[1, 1] / (cm[1, 0] + cm[1, 1])
specificity = cm[0, 0] / (cm[0, 0] + cm[0, 1])
```
6. 绘制ROC曲线:
```python
fpr, tpr, thresholds = roc_curve(y, y_pred)
plt.plot(fpr, tpr)
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC Curve')
plt.show()
```
请注意,上述代码中的`y`是真实标签值,`y_pred`是模型的预测结果。另外,混淆矩阵中的`cm[0, 0]`表示真实类别为负样本且被正确预测为负样本的数量,`cm[0, 1]`表示真实类别为负样本但被错误预测为正样本的数量,`cm[1, 0]`表示真实类别为正样本但被错误预测为负样本的数量,`cm[1, 1]`表示真实类别为正样本且被正确预测为正样本的数量。
以上是求解指标和绘制ROC曲线和混淆矩阵的基本步骤,你可以根据需要进一步调整和优化。
相关推荐
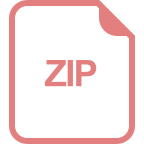












