Vue做一个有登录和注册界面 登录后里面有猜数字大小加留言板代码
时间: 2024-02-23 07:00:04 浏览: 27
好的,下面是一个简单的实现示例,您可以参考它来实现您的Vue应用程序。
首先,我们需要安装Vue.js和Vue Router。您可以使用以下命令在命令行中安装它们:
```
npm install vue
npm install vue-router
```
然后,您可以创建一个Vue实例,并在其中定义路由。以下是一个简单的示例:
```javascript
import Vue from 'vue'
import VueRouter from 'vue-router'
import Login from './components/Login.vue'
import Register from './components/Register.vue'
import Game from './components/Game.vue'
import Comments from './components/Comments.vue'
Vue.use(VueRouter)
const routes = [
{ path: '/login', component: Login },
{ path: '/register', component: Register },
{ path: '/game', component: Game },
{ path: '/comments', component: Comments }
]
const router = new VueRouter({
routes // short for `routes: routes`
})
new Vue({
router
}).$mount('#app')
```
在这个示例中,我们导入了四个组件,分别是Login、Register、Game和Comments。然后我们定义了四个路由,每个路由都对应一个组件。最后,我们创建了一个Vue实例,并将路由传递给Vue Router。
接下来,我们需要编写组件。以下是一个简单的示例:
```vue
<template>
<div>
<h1>Login</h1>
<form>
<label>Username:</label>
<input type="text" v-model="username">
<label>Password:</label>
<input type="password" v-model="password">
<button type="submit" @click.prevent="login">Login</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
username: '',
password: ''
}
},
methods: {
login() {
// Call login API here
// Redirect to game page
this.$router.push('/game')
}
}
}
</script>
```
在这个示例中,我们创建了一个Login组件,它包含一个表单,用户可以输入他们的用户名和密码。当用户点击登录按钮时,我们将调用一个API以验证用户凭据,然后将用户重定向到游戏页面。
接下来,我们需要编写一个Game组件,它实现猜数字大小游戏。以下是一个简单的示例:
```vue
<template>
<div>
<h1>Guess the Number</h1>
<p v-if="!started">Click the button to start the game!</p>
<div v-if="started">
<p v-if="guess === number">You win!</p>
<p v-else-if="guess < number">Too low!</p>
<p v-else>Too high!</p>
<form>
<label>Guess:</label>
<input type="number" v-model="guess">
<button type="submit" @click.prevent="makeGuess">Submit</button>
</form>
</div>
<button v-if="!started" @click="startGame">Start Game</button>
</div>
</template>
<script>
export default {
data() {
return {
started: false,
number: 0,
guess: 0
}
},
methods: {
startGame() {
this.started = true
this.number = Math.floor(Math.random() * 100) + 1
},
makeGuess() {
// Check the guess and update the message
}
}
}
</script>
```
在这个示例中,我们创建了一个Game组件,它包含一个按钮,用户可以点击它来开始游戏。当游戏开始时,我们将生成一个随机数,并显示一个表单,用户可以在其中猜测数值。当用户提交他们的猜测时,我们将检查它并更新消息,告诉用户他们的猜测是太低、太高还是正确的。
最后,我们需要编写一个Comments组件,它实现留言板。以下是一个简单的示例:
```vue
<template>
<div>
<h1>Comments</h1>
<form>
<label>Name:</label>
<input type="text" v-model="name">
<label>Comment:</label>
<textarea v-model="comment"></textarea>
<button type="submit" @click.prevent="submitComment">Submit</button>
</form>
<ul>
<li v-for="comment in comments">
<strong>{{ comment.name }}</strong>: {{ comment.comment }}
</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
name: '',
comment: '',
comments: []
}
},
methods: {
submitComment() {
// Add the comment to the list
this.comments.push({ name: this.name, comment: this.comment })
// Clear the form
this.name = ''
this.comment = ''
}
}
}
</script>
```
在这个示例中,我们创建了一个Comments组件,它包含一个表单,用户可以输入他们的名称和评论。当用户提交评论时,我们将将它添加到一个列表中,并清除表单。
希望这个示例能帮助您开始Vue开发。如果您有任何其他问题,请随时问我。
相关推荐
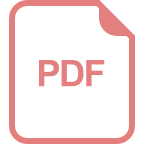
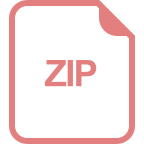














