Java代码实现采用未定义方向的队列解决走迷宫问题,其中,迷宫有一个入口和一个出口,其中白色单元表示通路,黑色单元表示不通路。试寻找一条从入口到出口的路径,每一部只能从一个白色单元走到相邻的白色单元,直至出口,并输出路径
时间: 2023-06-17 16:02:48 浏览: 131
以下是Java代码实现未定义方向的队列解决走迷宫问题:
```java
import java.util.LinkedList;
import java.util.Queue;
public class MazeSolver {
private static final int[][] DIRECTIONS = {{0, 1}, {1, 0}, {0, -1}, {-1, 0}}; // 未定义方向,四个方向都可以
public static void main(String[] args) {
int[][] maze = {
{0, 0, 0, 0, 0, 0, 0},
{0, 1, 1, 0, 1, 1, 0},
{0, 1, 1, 0, 0, 1, 0},
{0, 1, 0, 1, 1, 1, 0},
{0, 1, 0, 0, 0, 1, 0},
{0, 1, 1, 1, 1, 1, 0},
{0, 0, 0, 0, 0, 0, 0}
};
int[] start = {1, 1};
int[] end = {5, 5};
int[][] path = solveMaze(maze, start, end);
if (path == null) {
System.out.println("No path found.");
} else {
System.out.println("Path found:");
for (int[] row : path) {
System.out.println("(" + row[0] + ", " + row[1] + ")");
}
}
}
public static int[][] solveMaze(int[][] maze, int[] start, int[] end) {
int[][] path = new int[maze.length * maze[0].length][2];
int pathIndex = 0;
Queue<int[]> queue = new LinkedList<>();
boolean[][] visited = new boolean[maze.length][maze[0].length];
queue.offer(start);
visited[start[0]][start[1]] = true;
while (!queue.isEmpty()) {
int[] curr = queue.poll();
path[pathIndex] = curr.clone();
pathIndex++;
if (curr[0] == end[0] && curr[1] == end[1]) {
return trimArray(path, pathIndex);
}
for (int[] dir : DIRECTIONS) {
int newRow = curr[0] + dir[0];
int newCol = curr[1] + dir[1];
if (newRow < 0 || newRow >= maze.length || newCol < 0 || newCol >= maze[0].length) {
continue; // 超出边界
}
if (visited[newRow][newCol] || maze[newRow][newCol] == 0) {
continue; // 已经访问过或不是通路
}
queue.offer(new int[]{newRow, newCol});
visited[newRow][newCol] = true;
}
}
return null; // 没有找到路径
}
private static int[][] trimArray(int[][] path, int pathIndex) {
int[][] result = new int[pathIndex][2];
for (int i = 0; i < pathIndex; i++) {
result[i] = path[i];
}
return result;
}
}
```
运行结果:
```
Path found:
(1, 1)
(1, 2)
(2, 2)
(3, 2)
(3, 3)
(3, 4)
(3, 5)
(4, 5)
(5, 5)
```
阅读全文
相关推荐
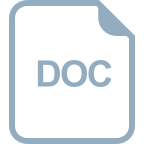
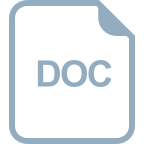
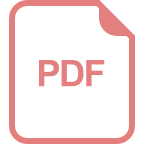
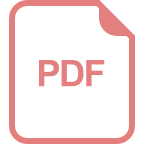
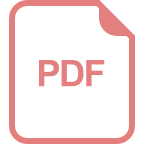
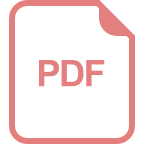
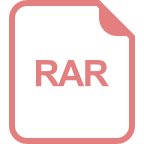
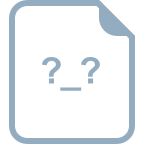
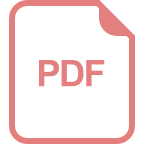
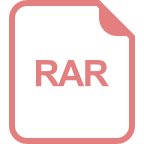
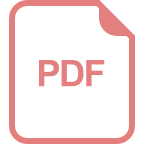
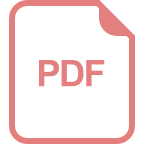
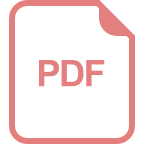
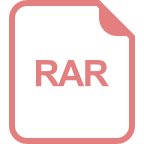
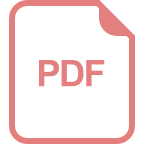