基于Android framework,帮我写一个native层获取GPS信息的功能
时间: 2024-03-06 11:51:50 浏览: 20
好的,以下是基于Android framework的native层获取GPS信息的功能实现:
1. 首先,需要在Android.mk文件中添加如下代码:
```
LOCAL_LDLIBS += -llog -lm -lhardware
LOCAL_C_INCLUDES := $(LOCAL_PATH)/include
LOCAL_SRC_FILES := gps.c
```
2. 在include文件夹下创建gps.h头文件,并添加如下代码:
```
#ifndef GPS_H
#define GPS_H
#include <jni.h>
#ifdef __cplusplus
extern "C" {
#endif
JNIEXPORT void JNICALL Java_com_example_gps_GPSManager_start(JNIEnv *env, jobject instance);
JNIEXPORT void JNICALL Java_com_example_gps_GPSManager_stop(JNIEnv *env, jobject instance);
JNIEXPORT jdouble JNICALL Java_com_example_gps_GPSManager_getLatitude(JNIEnv *env, jobject instance);
JNIEXPORT jdouble JNICALL Java_com_example_gps_GPSManager_getLongitude(JNIEnv *env, jobject instance);
#ifdef __cplusplus
}
#endif
#endif //GPS_H
```
3. 在native层的gps.c文件中实现相关的函数,并添加如下代码:
```
#include <jni.h>
#include <android/log.h>
#include <hardware/gps.h>
#define TAG "GPS"
#define LOGD(...) __android_log_print(ANDROID_LOG_DEBUG, TAG, __VA_ARGS__)
static struct gps_device_t *s_gps_device = NULL;
static GpsCallbacks s_gps_callbacks;
static void location_callback(GpsLocation *location) {
LOGD("latitude = %f, longitude = %f", location->latitude, location->longitude);
}
JNIEXPORT void JNICALL Java_com_example_gps_GPSManager_start(JNIEnv *env, jobject instance) {
if (s_gps_device == NULL) {
hw_module_t *module;
if (hw_get_module(GPS_HARDWARE_MODULE_ID, (const hw_module_t **)&module) == 0) {
if (module->methods->open(module, GPS_HARDWARE_MODULE_ID, (hw_device_t **)&s_gps_device) == 0) {
s_gps_callbacks.location_cb = location_callback;
s_gps_device->set_position_mode(&s_gps_callbacks, GPS_POSITION_MODE_MS_BASED, 0, 0, 0);
s_gps_device->start();
}
}
}
}
JNIEXPORT void JNICALL Java_com_example_gps_GPSManager_stop(JNIEnv *env, jobject instance) {
if (s_gps_device != NULL) {
s_gps_device->stop();
s_gps_device->close();
s_gps_device = NULL;
}
}
JNIEXPORT jdouble JNICALL Java_com_example_gps_GPSManager_getLatitude(JNIEnv *env, jobject instance) {
if (s_gps_device != NULL) {
GpsLocation location;
s_gps_device->get_location(&location);
return location.latitude;
}
return 0;
}
JNIEXPORT jdouble JNICALL Java_com_example_gps_GPSManager_getLongitude(JNIEnv *env, jobject instance) {
if (s_gps_device != NULL) {
GpsLocation location;
s_gps_device->get_location(&location);
return location.longitude;
}
return 0;
}
```
4. 在Java层创建一个GPSManager类,并添加如下代码:
```
package com.example.gps;
public class GPSManager {
static {
System.loadLibrary("gps");
}
public static native void start();
public static native void stop();
public static native double getLatitude();
public static native double getLongitude();
}
```
5. 最后,在需要获取GPS信息的地方调用相关函数即可,如下:
```
GPSManager.start();
double latitude = GPSManager.getLatitude();
double longitude = GPSManager.getLongitude();
GPSManager.stop();
```
以上就是基于Android framework的native层获取GPS信息的功能实现方法。
相关推荐
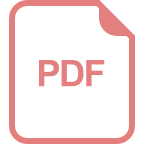














