<span>标签中的文字设置为居中
时间: 2024-03-11 13:46:48 浏览: 130
可以使用CSS样式将<span>标签中的文字设置为居中,如下所示:
```css
span {
text-align: center;
}
```
这样设置之后,<span>标签中的文字就会水平居中显示。如果需要垂直居中,还需要设置<span>标签的高度和行高相等,如下所示:
```css
span {
display: inline-block;
height: 30px;
line-height: 30px;
text-align: center;
}
```
这样设置之后,<span>标签中的文字就会水平垂直居中显示。
相关问题
请把一下代码每一行的用途注释上:<!DOCTYPE html> <html> <head> <meta charset="utf-8" /> <title>登录表单</title> <style> body { background-image: url("background.jpg"); background-size: cover; font-family: "宋体"; font-size: 30px; } form { margin: auto; width: 50%; background-color: rgba(255,255,255,0.8); padding: 20px; border-radius: 10px; } input[type=text], input[type=password] { width: 100%; padding: 12px 20px; margin: 8px 0; display: inline-block; border: 1px solid #ccc; border-radius: 4px; box-sizing: border-box; } input[type=submit], input[type=reset] { background-color: #4CAF50; color: white; padding: 14px 20px; margin: 8px 0; border: none; border-radius: 4px; cursor: pointer; } input[type=submit]:hover, input[type=reset]:hover { background-color: #45a049; } .error { color: red; } </style> </head> <body> <form> <h2>登录</h2> <label for="username">用户名</label> <input type="text" id="username" name="username" ><br> <label for="password">密码</label> <input type="password" id="password" name="password" ><br> <label for="confirm_password">确认密码</label> <input type="password" id="confirm_password" name="confirm_password" ><br> <input type="submit" value="登录"> <input type="reset" value="重置"> <span class="error" id="password_error"></span><br> <a href="https://www.baidu.com/">忘记密码?</a> </form> <script> document.querySelector("form").addEventListener("submit", function(event) { var password = document.querySelector("#password").value; var confirm_password = document.querySelector("#confirm_password").value; if (password != confirm_password) { document.querySelector("#password_error").innerHTML = "密码不一致"; event.preventDefault(); } }); </script> </body> </html>
```
<!DOCTYPE html> // 声明文档类型为 HTML5
<html> // HTML 根元素
<head> // 头部元素,包含了文档的元数据和链接到外部样式表和脚本文件等信息
<meta charset="utf-8" /> // 指定文档使用的字符编码为 UTF-8
<title>登录表单</title> // 设置文档的标题
<style> // 定义内部样式表
body { // 定义 body 元素的样式
background-image: url("background.jpg"); // 设置背景图片
background-size: cover; // 背景图片自适应元素大小
font-family: "宋体"; // 设置字体
font-size: 30px; // 设置字体大小
}
form { // 定义 form 元素的样式
margin: auto; // 居中显示
width: 50%; // 宽度为父元素的一半
background-color: rgba(255,255,255,0.8); // 背景颜色为白色,并设置透明度
padding: 20px; // 内边距为 20px
border-radius: 10px; // 设置边框圆角
}
input[type=text], input[type=password] { // 定义文本输入框和密码输入框的样式
width: 100%; // 宽度为父元素的 100%
padding: 12px 20px; // 内边距为 12px 和 20px
margin: 8px 0; // 外边距为 8px 和 0
display: inline-block; // 设置为行内块元素
border: 1px solid #ccc; // 设置边框
border-radius: 4px; // 设置边框圆角
box-sizing: border-box; // 设置盒子模型为 border-box
}
input[type=submit], input[type=reset] { // 定义提交按钮和重置按钮的样式
background-color: #4CAF50; // 设置背景颜色为绿色
color: white; // 设置文字颜色为白色
padding: 14px 20px; // 内边距为 14px 和 20px
margin: 8px 0; // 外边距为 8px 和 0
border: none; // 去掉边框
border-radius: 4px; // 设置边框圆角
cursor: pointer; // 鼠标指针为手型
}
input[type=submit]:hover, input[type=reset]:hover { // 鼠标悬停在提交按钮和重置按钮上时的样式
background-color: #45a049; // 背景颜色为深绿色
}
.error { // 定义错误提示信息的样式
color: red; // 设置文字颜色为红色
}
</style>
</head>
<body> // 文档的主体部分,包含了所有可见的内容
<form> // 定义一个表单
<h2>登录</h2> // 表单的标题
<label for="username">用户名</label> // 用户名输入框的标签
<input type="text" id="username" name="username" ><br> // 用户名输入框
<label for="password">密码</label> // 密码输入框的标签
<input type="password" id="password" name="password" ><br> // 密码输入框
<label for="confirm_password">确认密码</label> // 确认密码输入框的标签
<input type="password" id="confirm_password" name="confirm_password" ><br> // 确认密码输入框
<input type="submit" value="登录"> // 提交按钮
<input type="reset" value="重置"> // 重置按钮
<span class="error" id="password_error"></span><br> // 显示密码错误提示信息的元素
<a href="https://www.baidu.com/">忘记密码?</a> // 忘记密码的链接
</form>
<script> // 定义脚本
document.querySelector("form").addEventListener("submit", function(event) { // 监听表单的 submit 事件
var password = document.querySelector("#password").value; // 获取密码输入框的值
var confirm_password = document.querySelector("#confirm_password").value; // 获取确认密码输入框的值
if (password != confirm_password) { // 如果密码和确认密码不一致
document.querySelector("#password_error").innerHTML = "密码不一致"; // 显示错误提示信息
event.preventDefault(); // 阻止表单提交
}
});
</script>
</body>
</html>
```
html文件中 <td>使用rowspan="2"上下跨行,文字设置上下左右居中
在HTML中,`<td>` (表格数据单元格) 元素通常用于创建表格的内容。当需要在一个单元格跨越两行时,可以使用 `rowspan="2"` 属性。这会使得该单元格占据它下面一行的空间,并将内容显示在同一列里。
为了实现文字在跨越的两个单元格内的垂直及水平居中,你可以采用CSS样式来调整。首先,在`<table>`标签内添加一个内部样式,或者单独为这个单元格定义一个类名:
```html
<style>
.center-merged-cell {
display: flex; /* 使用 Flexbox 实现 */
align-items: center; /* 水平居中 */
justify-content: center; /* 垂直居中 */
vertical-align: middle; /* 为兼容老版本浏览器 */
}
</style>
<table>
<!-- 表格行 -->
<tr>
<td>正常单元格</td>
<td class="center-merged-cell rowspan="2">跨行单元格<span style="display: block; height: 0; overflow: hidden;">(隐藏高度)</span></td>
</tr>
<!-- 下一行 -->
<tr>
<!-- 可留空,因为已经由上方合并单元格覆盖了 -->
</tr>
</table>
```
这里的关键点是通过设置`display: flex`,然后用`align-items: center`和`justify-content: center`实现水平和垂直居中。同时,添加一个透明的`<span>`元素和`height: 0; overflow: hidden;`是为了避免实际文本影响到行高计算,从而达到真正的跨行效果。
阅读全文
相关推荐
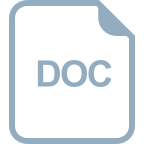
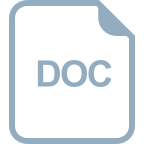
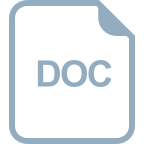






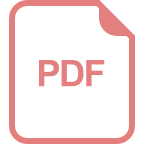






