java写一个实现对用户的增删查改 对商品的增删查改的购物系统
时间: 2024-06-08 20:07:10 浏览: 13
好的,这是一个比较常见的购物系统,我们可以使用Java语言来实现,以下是一个简单的代码示例:
```
import java.util.ArrayList;
import java.util.List;
class User {
String name;
String address;
String phoneNumber;
public User(String name, String address, String phoneNumber) {
this.name = name;
this.address = address;
this.phoneNumber = phoneNumber;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
public String getPhoneNumber() {
return phoneNumber;
}
public void setPhoneNumber(String phoneNumber) {
this.phoneNumber = phoneNumber;
}
}
class Product {
String name;
String description;
double price;
public Product(String name, String description, double price) {
this.name = name;
this.description = description;
this.price = price;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public double getPrice() {
return price;
}
public void setPrice(double price) {
this.price = price;
}
}
class ShoppingSystem {
List<User> users = new ArrayList<>();
List<Product> products = new ArrayList<>();
public void addUser(User user) {
users.add(user);
}
public void removeUser(User user) {
users.remove(user);
}
public User findUserByName(String name) {
for (User user : users) {
if (user.getName().equals(name)) {
return user;
}
}
return null;
}
public void addProduct(Product product) {
products.add(product);
}
public void removeProduct(Product product) {
products.remove(product);
}
public Product findProductByName(String name) {
for (Product product : products) {
if (product.getName().equals(name)) {
return product;
}
}
return null;
}
}
public class Main {
public static void main(String[] args) {
ShoppingSystem shoppingSystem = new ShoppingSystem();
User user1 = new User("张三", "上海市浦东新区", "13800000001");
User user2 = new User("李四", "上海市闵行区", "13800000002");
shoppingSystem.addUser(user1);
shoppingSystem.addUser(user2);
System.out.println(shoppingSystem.findUserByName("张三").getPhoneNumber());
Product product1 = new Product("手机", "苹果手机", 5000);
Product product2 = new Product("电脑", "戴尔电脑", 8000);
shoppingSystem.addProduct(product1);
shoppingSystem.addProduct(product2);
System.out.println(shoppingSystem.findProductByName("电脑").getPrice());
}
}
```
以上是一个简单的购物系统的Java实现,其中包含了对用户和商品的增删查改操作。当然这里只是一个简单的示例,实际系统中还需要考虑很多其他功能和细节。
相关推荐
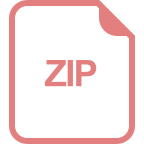
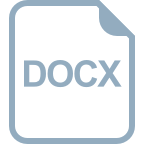
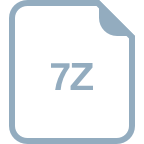
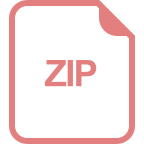