如何使用fxml设计出和网页版微信样式类似的页面?请给出具体代码
时间: 2024-03-18 11:44:49 浏览: 19
设计一个和网页版微信样式类似的页面可以使用JavaFX中的FXML来实现。以下是一个简单的示例代码,用于演示如何使用FXML实现一个类似的页面:
FXML文件(wechat.fxml):
```
<?xml version="1.0" encoding="UTF-8"?>
<?import javafx.scene.control.Label?>
<?import javafx.scene.control.ScrollPane?>
<?import javafx.scene.control.SplitPane?>
<?import javafx.scene.control.TextField?>
<?import javafx.scene.image.ImageView?>
<?import javafx.scene.layout.AnchorPane?>
<?import javafx.scene.layout.BorderPane?>
<?import javafx.scene.layout.HBox?>
<?import javafx.scene.layout.VBox?>
<BorderPane fx:id="root" prefHeight="600.0" prefWidth="800.0" xmlns="http://javafx.com/javafx/11.0.2" xmlns:fx="http://javafx.com/fxml/1">
<top>
<VBox prefHeight="60.0" prefWidth="800.0" style="-fx-background-color: #00bfff;">
<HBox spacing="10.0">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<Label text="John Smith" textFill="white" />
</HBox>
</VBox>
</top>
<center>
<SplitPane dividerPositions="0.3" prefHeight="540.0" prefWidth="800.0" style="-fx-border-color: #d9d9d9;">
<AnchorPane>
<ScrollPane fitToWidth="true" prefHeight="540.0" prefWidth="240.0" vbarPolicy="ALWAYS">
<VBox prefHeight="540.0" prefWidth="240.0" spacing="10.0">
<HBox spacing="10.0">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<Label text="Group 1" />
</HBox>
<HBox spacing="10.0">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<Label text="Group 2" />
</HBox>
<HBox spacing="10.0">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<Label text="Group 3" />
</HBox>
</VBox>
</ScrollPane>
</AnchorPane>
<AnchorPane>
<ScrollPane fitToWidth="true" prefHeight="540.0" prefWidth="560.0" vbarPolicy="ALWAYS">
<VBox prefHeight="540.0" prefWidth="560.0" spacing="10.0">
<HBox spacing="10.0">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<Label text="John Smith" />
<Label text="11:30 AM" />
</HBox>
<HBox spacing="10.0">
<Label style="-fx-background-color: #d9d9d9; -fx-border-radius: 10px;" text="Hello World!" wrapText="true" />
</HBox>
<HBox spacing="10.0">
<Label style="-fx-background-color: #00bfff; -fx-text-fill: white; -fx-border-radius: 10px;" text="Hi there!" wrapText="true" />
</HBox>
</VBox>
</ScrollPane>
<HBox prefHeight="60.0" prefWidth="560.0" style="-fx-background-color: #f5f5f5;">
<ImageView fitHeight="40.0" fitWidth="40.0" pickOnBounds="true" preserveRatio="true">
<image>
<Image url="@../images/avatar.jpg" />
</image>
</ImageView>
<TextField prefHeight="40.0" prefWidth="480.0" promptText="Type a message..." />
</HBox>
</AnchorPane>
</SplitPane>
</center>
</BorderPane>
```
Java文件(Main.java):
```
import javafx.application.Application;
import javafx.fxml.FXMLLoader;
import javafx.scene.Parent;
import javafx.scene.Scene;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) throws Exception{
Parent root = FXMLLoader.load(getClass().getResource("wechat.fxml"));
primaryStage.setTitle("WeChat");
primaryStage.setScene(new Scene(root, 800, 600));
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
```
在这个示例中,我们使用了BorderPane作为根节点,将页面分为了顶部和中心两个部分。顶部使用VBox和HBox布局来显示当前用户的头像和用户名。中心部分使用SplitPane将聊天列表和聊天记录分为了两个区域。聊天列表使用ScrollPane和VBox来显示所有的聊天组,聊天记录使用ScrollPane和VBox来显示所有的聊天消息,并使用HBox和Label来显示每条聊天消息的发送者、内容和时间。最后,我们使用HBox和TextField来实现聊天输入框。
相关推荐
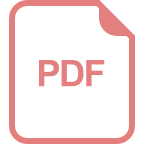
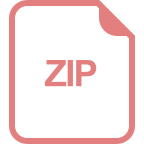
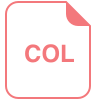
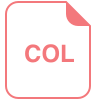
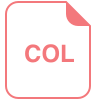
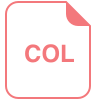
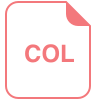









