对一幅带有噪声图像分别采用2种以上空域和频域的滤波方法实现平滑处理,空域和频域至少选择一种方法自己编写代码实现
时间: 2024-05-07 11:16:06 浏览: 12
以下是Python代码实现:
空域滤波:
```python
import cv2
import numpy as np
# 读取图像并加噪声
img = cv2.imread('lena.jpg', 0)
noise = np.random.normal(0, 50, img.shape)
noisy_img = np.clip(img + noise, 0, 255).astype(np.uint8)
# 定义均值滤波器
def mean_filter(img, kernel_size):
kernel = np.ones((kernel_size, kernel_size), np.float32) / (kernel_size ** 2)
filtered_img = cv2.filter2D(img, -1, kernel)
return filtered_img
# 定义中值滤波器
def median_filter(img, kernel_size):
filtered_img = cv2.medianBlur(img, kernel_size)
return filtered_img
# 使用均值滤波器和中值滤波器进行平滑处理
mean_filtered_img = mean_filter(noisy_img, 5)
median_filtered_img = median_filter(noisy_img, 5)
# 显示结果
cv2.imshow('Noisy Image', noisy_img)
cv2.imshow('Mean Filtered Image', mean_filtered_img)
cv2.imshow('Median Filtered Image', median_filtered_img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
频域滤波:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
# 读取图像并加噪声
img = cv2.imread('lena.jpg', 0)
noise = np.random.normal(0, 50, img.shape)
noisy_img = np.clip(img + noise, 0, 255).astype(np.uint8)
# 定义傅里叶变换函数
def fft(img):
f = np.fft.fft2(img)
fshift = np.fft.fftshift(f)
return fshift
# 定义傅里叶逆变换函数
def ifft(fshift):
f_ishift = np.fft.ifftshift(fshift)
img_back = np.fft.ifft2(f_ishift)
img_back = np.real(img_back)
return img_back
# 定义理想低通滤波器
def ideal_lpf(img, d):
rows, cols = img.shape
crow, ccol = int(rows / 2), int(cols / 2)
mask = np.zeros((rows, cols), np.uint8)
cv2.circle(mask, (ccol, crow), d, 1, -1)
fshift = fft(img)
fshift = fshift * mask
img_back = ifft(fshift)
img_back = np.clip(img_back, 0, 255).astype(np.uint8)
return img_back
# 定义高斯低通滤波器
def gaussian_lpf(img, d):
rows, cols = img.shape
crow, ccol = int(rows / 2), int(cols / 2)
x = np.arange(cols)
y = np.arange(rows)
x, y = np.meshgrid(x, y)
mask = np.exp(-((x - ccol) ** 2 + (y - crow) ** 2) / (2 * d ** 2))
fshift = fft(img)
fshift = fshift * mask
img_back = ifft(fshift)
img_back = np.clip(img_back, 0, 255).astype(np.uint8)
return img_back
# 使用理想低通滤波器和高斯低通滤波器进行平滑处理
ideal_lpf_img = ideal_lpf(noisy_img, 50)
gaussian_lpf_img = gaussian_lpf(noisy_img, 50)
# 显示结果
plt.subplot(2, 2, 1), plt.imshow(noisy_img, cmap='gray')
plt.title('Input Image'), plt.xticks([]), plt.yticks([])
plt.subplot(2, 2, 2), plt.imshow(ideal_lpf_img, cmap='gray')
plt.title('Ideal LPF Image'), plt.xticks([]), plt.yticks([])
plt.subplot(2, 2, 3), plt.imshow(gaussian_lpf_img, cmap='gray')
plt.title('Gaussian LPF Image'), plt.xticks([]), plt.yticks([])
plt.show()
```
相关推荐
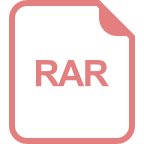
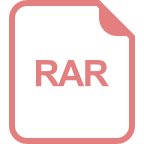
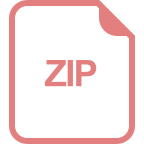














