android studio实现页面跳转
时间: 2023-04-24 18:01:56 浏览: 142
要在 Android Studio 中实现页面跳转,需要使用 Intent。Intent 是 Android 中用于跳转页面或启动其他应用组件的一种机制。可以使用 Intent 将用户从一个页面跳转到另一个页面。
示例代码:
```
// 创建 Intent 对象
Intent intent = new Intent(this, TargetActivity.class);
// 启动目标页面
startActivity(intent);
```
其中,this 是当前页面的上下文,TargetActivity 是要跳转到的目标页面。
还可以使用 Intent 传递数据,示例代码:
```
Intent intent = new Intent(this, TargetActivity.class);
intent.putExtra("key", value);
startActivity(intent);
```
在 TargetActivity 中可以使用 getIntent().getStringExtra("key") 或 getIntent().getIntExtra("key", defaultValue) 获取传递过来的值。
相关问题
Android Studio实现页面跳转
Android Studio实现页面跳转有两种方式可以选择。一种是通过MainActivity跳转,另一种是通过RelativeLayout布局跳转。
首先,我们可以在布局文件activity_main.xml中设置RelativeLayout,用于显示页面的内容。在这个布局中,可以添加各种视图组件,如TextView和Button。
第二步是在MainActivity.java文件中编写代码来实现页面跳转。您可以使用Intent类来启动另一个Activity,并在需要传递参数时使用Bundle对象。以下是使用MainActivity跳转的示例代码:
```java
// 导入需要的类
import android.content.Intent;
import android.os.Bundle;
public class MainActivity extends AppCompatActivity {
// ...
// 在某个事件触发时执行页面跳转
public void goToSecondActivity() {
// 创建Intent对象,指定当前Activity和目标Activity
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
// 如果需要传递参数,可以使用Bundle对象进行传递
Bundle bundle = new Bundle();
bundle.putString("key", "value");
intent.putExtras(bundle);
// 启动目标Activity
startActivity(intent);
}
}
```
请注意,这只是一个示例。根据您的具体需求,您可能需要做一些修改。
另一种实现页面跳转的方式是通过RelativeLayout布局中的点击事件来实现。您可以在activity_main.xml文件中为Button添加onClick属性,并在MainActivity.java文件中编写对应的方法来处理点击事件。
```java
// 导入需要的类
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
public class MainActivity extends AppCompatActivity {
// ...
// 在布局中定义的方法,用于处理点击事件
public void goToSecondActivity(View view) {
// 创建Intent对象,指定当前Activity和目标Activity
Intent intent = new Intent(MainActivity.this, SecondActivity.class);
// 如果需要传递参数,可以使用Bundle对象进行传递
Bundle bundle = new Bundle();
bundle.putString("key", "value");
intent.putExtras(bundle);
// 启动目标Activity
startActivity(intent);
}
}
```
请注意,这里的方法名和参数类型必须与布局文件中定义的onClick属性所指定的一致。
通过这两种方式,您可以在Android Studio中实现页面跳转。具体选择哪种方式取决于您的需求和设计。
android studio 实现页面跳转
在Android Studio中实现页面跳转可以通过以下步骤:
1. 创建目标页面:首先,在项目的`res/layout`目录下创建一个新的XML布局文件,用于定义目标页面的UI界面。
2. 创建目标页面的Activity类:在项目的`java`目录下创建一个新的Java类,用于实现目标页面的逻辑功能。该类需要继承自`AppCompatActivity`。
3. 在源页面中添加跳转代码:在源页面的按钮点击事件或其他触发事件中,使用`Intent`类创建一个新的意图,并指定目标页面的Activity类。例如:
```java
Intent intent = new Intent(SourceActivity.this, TargetActivity.class);
startActivity(intent);
```
4. 在AndroidManifest.xml中注册目标页面:打开项目的`AndroidManifest.xml`文件,在`<application>`标签内添加目标页面的Activity声明。例如:
```xml
<activity android:name=".TargetActivity"></activity>
```
这样,当源页面中的按钮被点击时,就会触发页面跳转,跳转到目标页面。
阅读全文
相关推荐
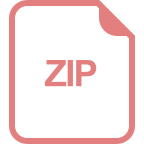


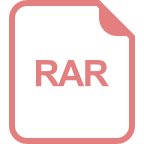










