java将文件放到一个实体类中返回给前端预览
时间: 2024-03-10 21:50:19 浏览: 25
要实现将文件放到一个实体类中返回给前端预览,你需要在实体类中添加一个方法,用于将文件内容转换成 Base64 编码的字符串,然后在返回实体类对象时将该字符串作为属性之一返回即可。
下面是示例代码:
```java
import org.springframework.util.StreamUtils;
import org.springframework.web.multipart.MultipartFile;
import java.io.IOException;
import java.io.InputStream;
import java.util.Base64;
public class FileEntity {
private String fileName;
private String fileType;
private String fileContent;
public FileEntity(MultipartFile file) throws IOException {
this.fileName = file.getOriginalFilename();
this.fileType = file.getContentType();
InputStream inputStream = file.getInputStream();
byte[] bytes = StreamUtils.copyToByteArray(inputStream);
this.fileContent = Base64.getEncoder().encodeToString(bytes);
}
public String getFileName() {
return fileName;
}
public String getFileType() {
return fileType;
}
public String getFileContent() {
return fileContent;
}
}
```
在控制器中,你只需要将上传的文件转换成 FileEntity 类对象并返回即可:
```java
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
@RestController
public class FileController {
@PostMapping("/upload")
public ResponseEntity<FileEntity> upload(@RequestParam("file") MultipartFile file) throws IOException {
FileEntity fileEntity = new FileEntity(file);
return new ResponseEntity<>(fileEntity, HttpStatus.OK);
}
}
```
在上面的示例代码中,上传文件的请求将会被映射到 /upload 路径。在 upload 方法中,我们将上传的文件转换成 FileEntity 类对象,并将该对象作为响应体返回给前端。在返回的 FileEntity 对象中,fileContent 属性的值是一个 Base64 编码的字符串,前端可以将其解码并使用相应的组件进行预览。
相关推荐
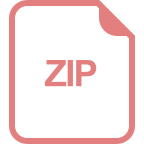
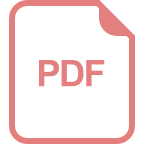
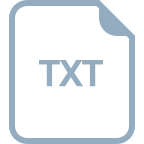














