python 凯撒密码加密hello world
时间: 2023-06-26 21:07:43 浏览: 67
以下是用 Python 实现的凯撒密码加密 "hello world":
```python
text = "hello world"
shift = 3
encrypted_text = ""
for char in text:
if char.isalpha():
# 获取字符的 ASCII 码值
ascii_code = ord(char)
# 根据偏移量 shift 计算出加密后的 ASCII 码值
encrypted_ascii = (ascii_code - 97 + shift) % 26 + 97
# 将加密后的 ASCII 码值转换为字符
encrypted_char = chr(encrypted_ascii)
encrypted_text += encrypted_char
else:
# 非字母字符不做加密处理
encrypted_text += char
print(encrypted_text) # 输出加密后的文本
```
输出结果为:"khoor zruog"
相关问题
python凯撒密码加密
凯撒密码加密是一种简单的密码加密方法,它基于字母表的移位,将原来的字母替换成偏移后的字母。
以下是一个Python示例代码,可以实现凯撒密码加密:
```python
def caesar_cipher_encrypt(plaintext, key):
ciphertext = ''
for char in plaintext:
if char.isalpha():
# 将字母移位
shifted_char = chr((ord(char) - ord('a') + key) % 26 + ord('a'))
ciphertext += shifted_char
else:
ciphertext += char
return ciphertext
```
代码中的`plaintext`是要加密的明文,`key`是移位的偏移量。首先遍历明文中的每个字符,如果是字母,则进行移位加密,否则直接添加到密文中。移位加密的方法是:将字符转换为ASCII码,减去字母a的ASCII码,加上偏移量,再对26取模,最后加上字母a的ASCII码,转换回字符。
以下是一个示例,将明文`hello world`使用偏移量为3的凯撒密码加密:
```python
plaintext = 'hello world'
key = 3
ciphertext = caesar_cipher_encrypt(plaintext, key)
print(ciphertext) # 输出:khoor zruog
```
密文为`khoor zruog`。
python凯撒密码加密算法
凯撒密码是一种古老的加密算法,它通过将明文中的每个字母按照一定的规则替换成密文中的字母来实现加密。下面是使用Python实现凯撒密码加密算法的代码:
```python
def caesar_encrypt(plaintext, key):
ciphertext = ""
for char in plaintext:
if char.isalpha():
if char.isupper():
ciphertext += chr((ord(char) + key - 65) % 26 + 65)
else:
ciphertext += chr((ord(char) + key - 97) % 26 + 97)
else:
ciphertext += char
return ciphertext
```
其中,`plaintext`是要加密的明文,`key`是密钥,表示每个字母向前移动的位数。这个函数会返回加密后的密文。
下面是一个例子,演示如何使用这个函数加密一个字符串:
```python
plaintext = "Hello, World!"
key = 3
ciphertext = caesar_encrypt(plaintext, key)
print(ciphertext) # 输出:Khoor, Zruog!
```
这个例子中,我们将明文字符串`"Hello, World!"`加密成了密文字符串`"Khoor, Zruog!"`,密钥为3。
相关推荐
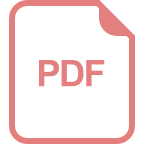
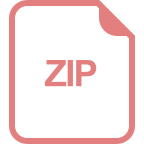
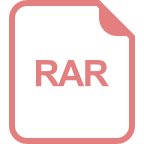
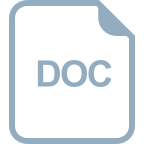
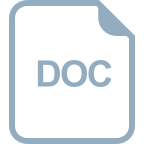
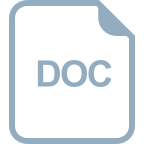
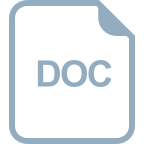
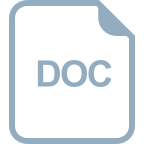
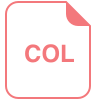
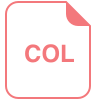
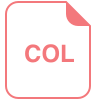
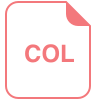
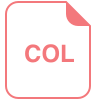


