帮我在python里面用baidu-aip和opencv库写一个可以先识别文件夹里面的照片的人脸信息,然后打开摄像头,如果镜头中出现人脸就进行比较判断,如果是同一个人就保存截图,如果不是就继续等待,用代码写出来
时间: 2023-04-11 15:01:49 浏览: 71
好的,以下是代码:
```python
import os
import cv2
from aip import AipFace
# 设置百度AI的参数
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
client = AipFace(APP_ID, API_KEY, SECRET_KEY)
# 设置摄像头
cap = cv2.VideoCapture(0)
# 设置人脸识别参数
options = {
'max_face_num': 1, # 最多检测一张人脸
'face_fields': 'age,beauty,gender' # 返回年龄、颜值和性别信息
}
# 读取文件夹中的照片并提取人脸信息
face_infos = []
for filename in os.listdir('path/to/folder'):
if filename.endswith('.jpg'):
filepath = os.path.join('path/to/folder', filename)
with open(filepath, 'rb') as f:
image = f.read()
result = client.detect(image, options)
if 'result' in result and 'face_list' in result['result'] and len(result['result']['face_list']) > 0:
face_info = result['result']['face_list'][0]
face_info['filepath'] = filepath
face_infos.append(face_info)
# 开始实时检测摄像头中的人脸
while True:
ret, frame = cap.read()
if not ret:
break
# 检测人脸
image = cv2.imencode('.jpg', frame)[1].tostring()
result = client.detect(image, options)
if 'result' in result and 'face_list' in result['result'] and len(result['result']['face_list']) > 0:
face_info = result['result']['face_list'][0]
# 判断是否为已知人脸
for known_face_info in face_infos:
if abs(face_info['age'] - known_face_info['age']) < 5 and abs(face_info['beauty'] - known_face_info['beauty']) < 10 and face_info['gender']['type'] == known_face_info['gender']['type']:
# 保存截图
cv2.imwrite('path/to/save/screenshot.jpg', frame)
break
# 显示摄像头画面
cv2.imshow('frame', frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头并关闭窗口
cap.release()
cv2.destroyAllWindows()
```
请注意,这只是一个简单的示例代码,实际应用中可能需要更复杂的人脸识别算法来提高准确率。
阅读全文
相关推荐
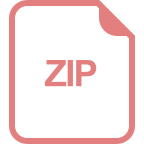
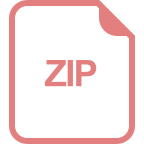
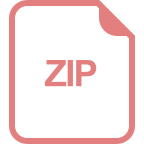


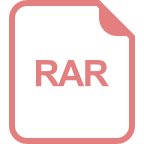
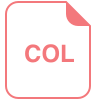

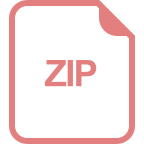
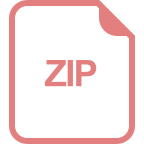
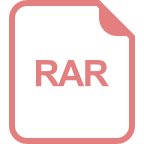
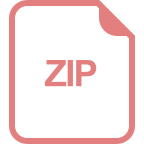
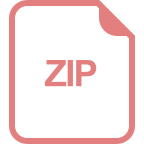
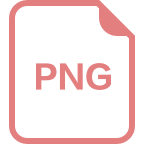