DFS求解八数码问题的代码,包含输出路径
时间: 2023-09-20 15:06:14 浏览: 108
### 回答1:
八数码问题可以使用深度优先搜索(DFS)来求解,以下是一个基于递归实现的DFS求解八数码问题的代码,包含输出路径。
```python
# 定义目标状态
target_state = [[1, 2, 3], [4, 5, 6], [7, 8, 0]]
# 计算当前状态与目标状态的曼哈顿距离
def manhattan_distance(state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
x, y = divmod(state[i][j] - 1, 3)
distance += abs(x - i) + abs(y - j)
return distance
# 深度优先搜索
def dfs(state, path, depth, max_depth):
if state == target_state:
print(path)
return True
if depth == max_depth:
return False
zero_x, zero_y = 0, 0
for i in range(3):
for j in range(3):
if state[i][j] == 0:
zero_x, zero_y = i, j
break
for dx, dy in ((0, 1), (1, 0), (0, -1), (-1, 0)):
x, y = zero_x + dx, zero_y + dy
if 0 <= x < 3 and 0 <= y < 3:
new_state = [row[:] for row in state]
new_state[zero_x][zero_y], new_state[x][y] = new_state[x][y], new_state[zero_x][zero_y]
if manhattan_distance(new_state) + depth <= max_depth and dfs(new_state, path + [(x, y)], depth + 1, max_depth):
return True
return False
# 初始状态
initial_state = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
# 逐层增加深度,直到找到解
for depth in range(1, 100):
if dfs(initial_state, [], 0, depth):
break
```
在这个算法中,我们使用曼哈顿距离来计算当前状态与目标状态的距离。每次选择一个空格的相邻格子进行交换,得到一个新的状态,并递归处理这个新状态。如果递归到达了指定的深度而没有找到解,则返回False。如果找到了解,则返回True并输出路径。
注意,由于八数码问题的解空间非常大,因此这个算法可能会产生很多无用的搜索。可以考虑使用剪枝等优化方法来提高效率。
### 回答2:
八数码问题是一个经典的搜索问题,可以用深度优先搜索(DFS)来解决。下面是一个利用DFS求解八数码问题的代码,并在其中包含了输出路径。
```python
# 定义八数码问题的初始状态和目标状态
initial_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
target_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
# 定义八数码问题的移动操作
# 数字0表示空格,可以与上下左右的数字进行交换
movements = [(0, 1), (0, -1), (1, 0), (-1, 0)]
movements_str = ['R', 'L', 'D', 'U']
# 判断当前状态是否是目标状态
def is_target_state(state):
return state == target_state
# 深度优先搜索
def dfs_search(state, path):
if is_target_state(state):
return path
for i in range(3):
for j in range(3):
if state[i][j] == 0:
for move, move_str in zip(movements, movements_str):
x, y = i + move[0], j + move[1]
if 0 <= x < 3 and 0 <= y < 3:
new_state = [row.copy() for row in state]
new_state[i][j], new_state[x][y] = new_state[x][y], new_state[i][j]
new_path = path + move_str
result = dfs_search(new_state, new_path)
if result is not None:
return result
return None
# 调用DFS算法求解八数码问题
result_path = dfs_search(initial_state, "")
if result_path is None:
print("无解")
else:
print("求解路径为:", result_path)
```
以上代码会从初始状态开始,通过深度优先搜索不断尝试各种移动操作,直到找到目标状态或者所有可能的路径都已经尝试过。求解路径的方式是用一个字符串记录每一步的移动操作(例如'R'表示向右移动),最终输出求解路径。如果无解,则输出"无解"。
### 回答3:
以下是使用深度优先搜索(DFS)算法求解八数码问题的代码,包含输出路径。
```python
# 定义状态类
class State:
def __init__(self, board, last_move, depth):
self.board = board # 当前的棋盘状态
self.last_move = last_move # 上一次移动的方向
self.depth = depth # 当前的搜索深度
def is_goal(self):
# 判断是否为目标状态
goal_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
return self.board == goal_state
def get_next_states(self):
# 获取下一步的所有可能状态
next_states = []
zero_i, zero_j = -1, -1
# 找到空格的位置
for i in range(3):
for j in range(3):
if self.board[i][j] == 0:
zero_i, zero_j = i, j
break
# 尝试进行上下左右的移动
moves = [(-1, 0), (1, 0), (0, -1), (0, 1)]
directions = ['上', '下', '左', '右']
for move, direction in zip(moves, directions):
new_board = [row[:] for row in self.board] # 复制当前棋盘状态
move_i, move_j = move
new_i, new_j = zero_i + move_i, zero_j + move_j
if 0 <= new_i < 3 and 0 <= new_j < 3:
# 如果移动合法,则交换空格和新位置的数字
new_board[zero_i][zero_j], new_board[new_i][new_j] = new_board[new_i][new_j], new_board[zero_i][zero_j]
next_states.append(State(new_board, direction, self.depth + 1))
return next_states
# 定义DFS函数
def dfs(start_state):
stack = [start_state] # 使用栈来存储待搜索的状态
visited = set() # 存储已经搜索过的状态
while stack:
current_state = stack.pop() # 弹出栈顶状态
if current_state.is_goal():
# 找到了目标状态,输出路径
path = []
while current_state:
path.append(current_state)
current_state = current_state.parent
print("移动步骤如下:")
for i in range(len(path)-1, -1, -1):
print("第{}步:{}".format(path[i].depth, path[i].last_move))
return
if current_state not in visited:
# 当前状态未访问过,加入visited集合
visited.add(current_state)
# 获取下一步的所有可能状态
next_states = current_state.get_next_states()
for next_state in next_states:
next_state.parent = current_state # 设置父状态
stack.append(next_state) # 将下一步状态加入栈中
print("未找到解答!")
# 使用示例
start_board = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
start_state = State(start_board, None, 0)
dfs(start_state)
```
以上代码通过在深度优先搜索过程中不断搜索下一步的所有可能状态,并使用栈来保存待搜索的状态。当找到目标状态时,通过存储状态的父状态,回溯输出移动路径。如果无法找到目标状态,则输出提示信息。
阅读全文
相关推荐


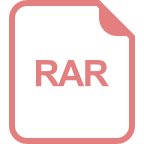
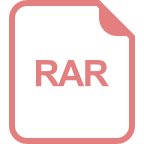




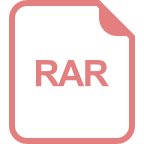



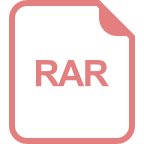



