if __name__ == '__main__': ep_robot = robot.Robot() ep_robot.initialize(conn_type="ap") ep_camera = ep_robot.camera为什么要这么写这段代码
时间: 2023-11-23 14:07:28 浏览: 31
此段代码是Python的一种惯用写法,主要是用来判断当前文件是被直接运行还是被其他文件导入的。在这段代码中,如果当前文件是直接运行的,则会执行后面的代码,而如果是被其他文件导入的,则不会执行后面的代码。这样做的好处是可以让一个文件既可以被当做脚本直接运行,又可以作为一个模块被其他文件导入使用。至于为什么要这样写,这主要是Python的一种约定俗成的写法。
相关问题
import pygame import sys from pygame.locals import * from robomaster import * import cv2 if __name__ == '__main__': # 如果本地IP 自动获取不正确,手动指定本地IP地址 # robomaster.config.LOCAL_IP_STR = "192.168.2.20" ep_robot = robot.Robot() # 指定连接方式为AP 直连模式 ep_robot.initialize(conn_type='ap') version = ep_robot.get_version() print("Robot version: {0}".format(version)) def show_video(): # 获取机器人第一视角图像帧 img = ep_robot.camera.read_cv2_image(strategy="newest") # 转换图像格式,转换为pygame的surface对象 # if img.any(): img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img = cv2.transpose(img) # 行列互换 img = pygame.surfarray.make_surface(img) screen.blit(img, (0, 0)) # 绘制图象 pygame.init() screen_size = width, height = 1280, 720 screen = pygame.display.set_mode(screen_size) ep_robot.camera.start_video_stream(display=False) pygame.time.wait(100) ep_robot.led.set_led(comp="all", r=255, g=255, b=255) # 亮绿灯 clock = pygame.time.Clock() while True: clock.tick(25) # 将帧数设置为25帧 for event in pygame.event.get(): if event.type == QUIT: ep_robot.close() pygame.quit() sys.exit() show_video() pygame.display.update()
这段代码是一个使用pygame和robomaster库进行机器人控制显示视频的示例代码。代码中首先导入了所需的库,然后创建了一个RobMaster机器人实例初始化连接方式为AP连模式。接下来获取机器人的版本信息并打印出来。
然后定义了一个名为show_video的函数,用于显示机器人的第一视角图像。在函数中,首先从机器人摄像头读取最新的图像帧,并转换为pygame的surface对象。然后将图像绘制到屏幕上。
着使用pygame进行初始化,并设置屏幕大小为1280x720。然后启动机器人的视频流,并等待100毫秒。同时,设置机器人的LED灯为全亮白色。
接下来进入主循环,其中使用clock对象设置帧率为25帧。在循环中,处理pygame的事件,当检测到QUIT事件时,关闭机器人连接、退出pygame并终止程序。然后调用show_video函数显示机器人视频,并更新屏幕显示。循环会不断重复执行,直到程序被终止。
请注意,这只是代码的一部分,可能还有其他部分未包含在内。如果你有关于这段代码的任何问题,请随时提问。
修改此代码使其可重复运行import pygame import sys from pygame.locals import * from robomaster import * import cv2 import numpy as np focal_length = 750 # 焦距 known_radius = 2 # 已知球的半径 def calculate_distance(focal_length, known_radius, perceived_radius): distance = (known_radius * focal_length) / perceived_radius return distance def show_video(ep_robot, screen): 获取机器人第一视角图像帧 img = ep_robot.camera.read_cv2_image(strategy="newest") 转换图像格式,转换为pygame的surface对象 img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) img = cv2.transpose(img) # 行列互换 img = pygame.surfarray.make_surface(img) screen.blit(img, (0, 0)) # 绘制图像 def detect_white_circle(ep_robot): 获取机器人第一视角图像帧 img = ep_robot.camera.read_cv2_image(strategy="newest") 转换为灰度图像 gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) 进行中值滤波处理 gray = cv2.medianBlur(gray, 5) 检测圆形轮廓 circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 50, param1=160, param2=40, minRadius=5, maxRadius=60) if circles is not None: circles = np.uint16(np.around(circles)) for circle in circles[0, :]: center = (circle[0], circle[1]) known_radius = circle 在图像上绘制圆形轮廓 cv2.circle(img, center, known_radius, (0, 255, 0), 2) 显示图像 distance = calculate_distance(focal_length, known_radius, known_radius) 在图像上绘制圆和距离 cv2.circle(img, center, known_radius, (0, 255, 0), 2) cv2.putText(img, f"Distance: {distance:.2f} cm", (center[0] - known_radius, center[1] - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2) cv2.imshow("White Circle Detection", img) cv2.waitKey(1) def main(): pygame.init() screen_size = width, height = 1280, 720 screen = pygame.display.set_mode(screen_size) ep_robot = robot.Robot() ep_robot.initialize(conn_type='ap') version = ep_robot.get_version() print("Robot version: {0}".format(version)) ep_robot.camera.start_video_stream(display=False) pygame.time.wait(100) clock = pygame.time.Clock() while True: clock.tick(5) # 将帧数设置为25帧 for event in pygame.event.get(): if event.type == QUIT: ep_robot.close() pygame.quit() sys.exit() show_video(ep_robot, screen) detect_white_circle(ep_robot) if name == 'main': main()
修改后的代码如下所示:
```python
import pygame
import sys
from pygame.locals import *
from robomaster import robot
import cv2
import numpy as np
focal_length = 750 # 焦距
known_radius = 2 # 已知球的半径
def calculate_distance(focal_length, known_radius, perceived_radius):
distance = (known_radius * focal_length) / perceived_radius
return distance
def show_video(ep_robot, screen):
# 获取机器人第一视角图像帧
img = ep_robot.camera.read_cv2_image(strategy="newest")
# 转换图像格式,转换为pygame的surface对象
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.transpose(img) # 行列互换
img = pygame.surfarray.make_surface(img)
screen.blit(img, (0, 0)) # 绘制图像
def detect_white_circle(ep_robot):
# 获取机器人第一视角图像帧
img = ep_robot.camera.read_cv2_image(strategy="newest")
# 转换为灰度图像
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 进行中值滤波处理
gray = cv2.medianBlur(gray, 5)
# 检测圆形轮廓
circles = cv2.HoughCircles(gray, cv2.HOUGH_GRADIENT, 1, 50, param1=160, param2=40, minRadius=5, maxRadius=60)
if circles is not None:
circles = np.uint16(np.around(circles))
for circle in circles[0, :]:
center = (circle[0], circle[1])
known_radius = circle[2]
# 在图像上绘制圆形轮廓
cv2.circle(img, center, known_radius, (0, 255, 0), 2)
# 计算距离
distance = calculate_distance(focal_length, known_radius, known_radius)
# 在图像上绘制圆和距离
cv2.circle(img, center, known_radius, (0, 255, 0), 2)
cv2.putText(img, f"Distance: {distance:.2f} cm", (center[0] - known_radius, center[1] - 10),
cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow("White Circle Detection", img)
cv2.waitKey(1)
def main():
pygame.init()
screen_size = width, height = 1280, 720
screen = pygame.display.set_mode(screen_size)
ep_robot = robot.Robot()
ep_robot.initialize(conn_type='ap')
version = ep_robot.get_version()
print("Robot version: {0}".format(version))
ep_robot.camera.start_video_stream(display=False)
pygame.time.wait(100)
clock = pygame.time.Clock()
while True:
clock.tick(5) # 将帧数设置为25帧
for event in pygame.event.get():
if event.type == QUIT:
ep_robot.close()
pygame.quit()
sys.exit()
show_video(ep_robot, screen)
detect_white_circle(ep_robot)
if __name__ == '__main__':
main()
```
相关推荐
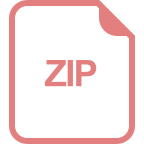






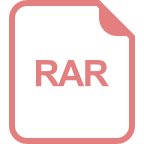