使用字典的方法输入日期判断第几天python
时间: 2024-02-28 07:24:16 浏览: 67
下面是一个使用字典的方法来判断日期是一年中的第几天的Python程序:
```python
def is_leap_year(year):
"""判断是否为闰年"""
return year % 4 == 0 and year % 100 != 0 or year % 400 == 0
def day_of_year(year, month, day):
"""计算日期是一年中的第几天"""
days_of_month = {1: 31, 2: 28, 3: 31, 4: 30, 5: 31, 6: 30, 7: 31, 8: 31, 9: 30, 10: 31, 11: 30, 12: 31}
if is_leap_year(year):
days_of_month[2] = 29
days = 0
for i in range(1, month):
days += days_of_month[i]
days += day
return days
# 测试
print(day_of_year(2020, 3, 1)) # 61
print(day_of_year(2021, 5, 1)) # 121
```
以上程序中,我们定义了一个 `is_leap_year()` 函数来判断闰年。然后,我们使用一个字典来存储每个月的天数,如果是闰年,则将二月的天数修改为 29。最后,我们遍历月份计算出前几个月的天数之和,再加上本月的天数即可得到日期是一年中的第几天。
相关问题
使用字典实现python中输入日期判断是第几天
以下是使用字典实现输入日期判断是第几天的Python代码:
```
def is_leap(year):
return year % 4 == 0 and (year % 100 != 0 or year % 400 == 0)
def day_of_year(date):
year, month, day = map(int, date.split('-'))
days_in_month = {1: 31, 2: 28, 3: 31, 4: 30, 5: 31, 6: 30, 7: 31, 8: 31, 9: 30, 10: 31, 11: 30, 12: 31}
if is_leap(year):
days_in_month[2] = 29
day_count = 0
for i in range(1, month):
day_count += days_in_month[i]
day_count += day
return day_count
date = input('请输入日期(格式为YYYY-MM-DD):')
day = day_of_year(date)
print(f'{date}是{day}年的第{day}天。')
```
该代码定义了两个函数,`is_leap(year)`用于判断给定年份是否是闰年,`day_of_year(date)`用于计算给定日期是该年的第几天。在`day_of_year(date)`函数中,使用了一个字典`days_in_month`,其中键为月份,值为该月的天数。如果给定年份是闰年,则将二月的天数修改为29。接着,使用一个循环遍历1到给定月份之前的所有月份,累加每个月的天数。最后,将累加的天数加上给定日期的天数,即可得到该日期是该年的第几天。最后,使用`input()`函数获取用户输入的日期,调用`day_of_year(date)`函数计算天数并输出结果。
python 输入某年某月某日,判断这一天是一年中的第几天 用字典解决
好的,可以使用字典来解决这个问题。下面是 Python 代码实现:
```python
# 定义月份天数的字典
month_days = {
1: 31,
2: 28,
3: 31,
4: 30,
5: 31,
6: 30,
7: 31,
8: 31,
9: 30,
10: 31,
11: 30,
12: 31
}
# 获取用户输入的日期
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 判断是否为闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
month_days[2] = 29
# 计算天数
days = sum([month_days[i] for i in range(1, month)]) + day
# 输出结果
print("这是{}年的第{}天。".format(year, days))
```
这个程序可以让用户输入一个日期,然后判断这个日期是这一年中的第几天。它使用了一个字典来存储每个月份的天数,然后根据用户输入的日期计算出这是这一年中的第几天。同时,它还考虑了闰年的情况,如果用户输入的年份是闰年,那么2月份的天数就应该是29天。
阅读全文
相关推荐
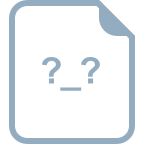
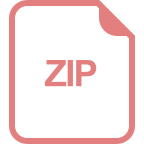
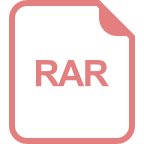
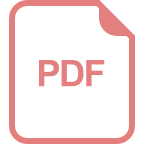
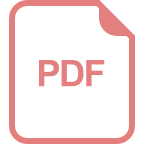
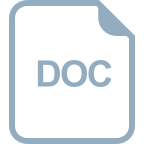
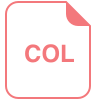
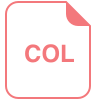
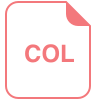
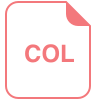
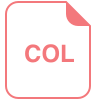
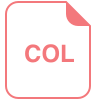
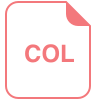
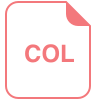
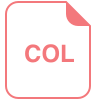