// 打印前 n 个出现次数最多的单词 void print_top_n(struct tnode** top_n, int n) { printf("Top %d words:\n", n); int i,j; for ( i = 0; i < n; i++) { if (top_n[i]->num == 0) { break; } printf("%d: ", i + 1); struct tnode* cur = top_n[i]; char word[200]={'\0'}; int len = 0; // 从 Trie 树中回溯到根节点,构造出单词 while (cur != NULL) { word[len++] = 'a' + (cur - cur->ptr[0]); cur = cur->parent; } for ( j = len - 2; j >= 0; j--) { printf("%c", word[j]); } printf(" (%d occurrences)\n", top_n[i]->num); } }优化这段代码
时间: 2023-12-14 09:40:07 浏览: 30
这段代码的主要瓶颈在于从 Trie 树中回溯到根节点,构造出单词的操作。可以使用一个字符数组来保存当前的单词,避免反复申请和释放内存。同时,还可以将从 Trie 树中回溯到根节点的操作改为使用指针数组进行,提高代码的可读性和执行效率。优化后的代码如下:
```
void print_top_n(struct tnode** top_n, int n) {
printf("Top %d words:\n", n);
for (int i = 0; i < n && top_n[i]->num > 0; ++i) {
printf("%d: ", i + 1);
struct tnode* cur = top_n[i];
char word[200];
int len = 0;
// 从 Trie 树中回溯到根节点,构造出单词
while (cur->parent != NULL) {
word[len++] = 'a' + (cur - cur->ptr[0]);
cur = cur->parent;
}
// 将单词翻转回来
for (int j = len - 1; j >= 0; --j) {
printf("%c", word[j]);
}
printf(" (%d occurrences)\n", top_n[i]->num);
}
}
```
相关推荐
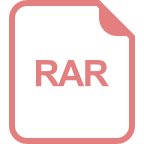















